


How does Expression SFINAE (Substitution Failure Is Not An Error) work in C and how can it be used to define traits and conditionally apply function overloads?
Understanding Expression SFINAE
In the context of C programming, Expression SFINAE (Substitution Failure Is Not An Error) allows you to conditionally apply function overloads based on the validity of an expression within the function declaration.
Expression SFINAE in Action
Consider the following code examples:
Example 1:
template <int I> struct A {}; char xxx(int); char xxx(float); template <class T> A<sizeof(xxx((T)0))> f(T) {}
The f() function returns a struct A with a parameter I set to the size of the result of calling xxx() with the casted value of the template parameter T to (T)0.
Example 2:
struct X {}; struct Y { Y(X) {} }; template <class T> auto f(T t1, T t2) -> decltype(t1 + t2); // #1 X f(Y, Y); // #2 X x1, x2; X x3 = f(x1, x2); // deduction fails on #1 (cannot add X+X), calls #2
In example 2, the f() function overloads allow for both addition of numerical types (#1) and construction of Y from X (#2). When the f() function is called with x1 and x2 (which are X objects), the overload that constructs Y is selected because the first overload is invalid for X objects.
Common Use Case: Trait Definition
Expression SFINAE is commonly used in trait definition, where it allows you to define traits based on the existence of specific member functions in a class. For example:
struct has_member_begin_test { template <class U> static auto test(U* p) -> decltype(p->begin(), std::true_type()); template <class> static auto test(...) -> std::false_type; }; template <class T> struct has_member_begin : decltype(has_member_begin_test::test<T>(0)) {};
The has_member_begin struct template can be used to check if a class has a begin() member function. It uses Expression SFINAE to determine if the begin() expression returns a valid type or expression, and returns std::true_type if valid, otherwise std::false_type.
Important Note:
Expression SFINAE is a relatively recent addition to the C language, and not all compilers fully support it. If you encounter any issues with Expression SFINAE in your code, it is important to verify that your compiler version supports it.
The above is the detailed content of How does Expression SFINAE (Substitution Failure Is Not An Error) work in C and how can it be used to define traits and conditionally apply function overloads?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










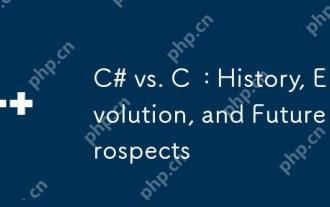
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
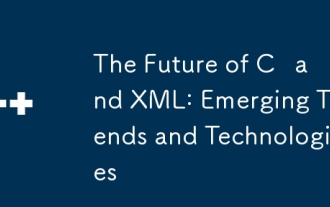
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
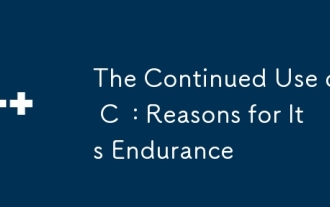
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
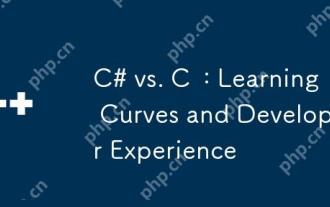
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
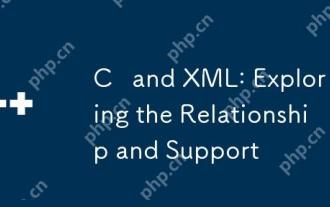
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
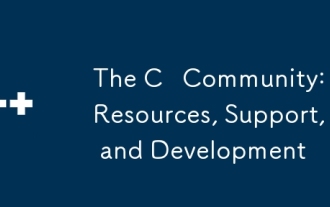
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
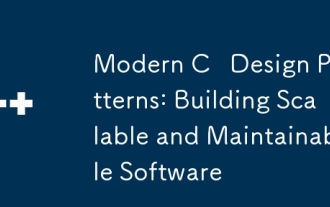
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
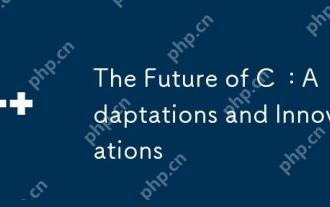
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
