How to Capture and Log HTTP Servlet Response Content in Java?
How to Capture and Log HTTP Servlet Response Content
Introduction
Logging both request and response content is essential for comprehensive server-side debugging and monitoring. While accessing the request parameters through a filter is straightforward, retrieving the response content can be more challenging. This article explains how to create a filter that intercepts and copies the servlet response for logging purposes.
Creating a Filter to Capture Response Content
To capture the response content, we need to create a custom Filter that wraps the HttpServletResponse object and overrides the getOutputStream() and getWriter() methods. These methods are responsible for providing streams to write response data. By overriding them, we can create a custom stream that intercepts the data and copies it into a buffer.
Here's an example of a filter that accomplishes this task:
@WebFilter("/*") public class ResponseLogger implements Filter { @Override public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) { HttpServletResponseCopier responseCopier = new HttpServletResponseCopier((HttpServletResponse) response); try { chain.doFilter(request, responseCopier); responseCopier.flushBuffer(); } finally { byte[] copy = responseCopier.getCopy(); System.out.println(new String(copy, response.getCharacterEncoding())); } } }
Customizing the Response Wrapper
The HttpServletResponseCopier class wraps the original HttpServletResponse and creates a custom ServletOutputStream that intercepts the data. The overridden getOutputStream() and getWriter() methods create and return the ServletOutputStreamCopier instance.
public class HttpServletResponseCopier extends HttpServletResponseWrapper { private ServletOutputStreamCopier copier; @Override public ServletOutputStream getOutputStream() { if (copier == null) { copier = new ServletOutputStreamCopier(getResponse().getOutputStream()); } return copier; } @Override public PrintWriter getWriter() { if (copier == null) { copier = new ServletOutputStreamCopier(getResponse().getOutputStream()); } return new PrintWriter(new OutputStreamWriter(copier, getResponse().getCharacterEncoding()), true); } }
Intercepting and Copying Response Data
The ServletOutputStreamCopier class overrides the write(int b) method to intercept the data written to the response. It writes the data to the original stream while also copying it into a ByteArrayOutputStream for later retrieval.
public class ServletOutputStreamCopier extends ServletOutputStream { private ByteArrayOutputStream copy; @Override public void write(int b) { outputStream.write(b); copy.write(b); } public byte[] getCopy() { return copy.toByteArray(); } }
Retrieving the Captured Response
After the request-response cycle has completed, the filter retrieves the captured response content from the ServletOutputStreamCopier's getCopy() method. This data can then be used for logging or any other desired purpose.
The above is the detailed content of How to Capture and Log HTTP Servlet Response Content in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










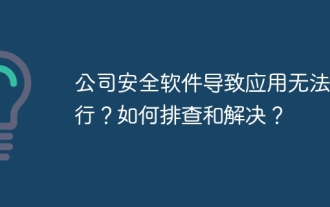
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
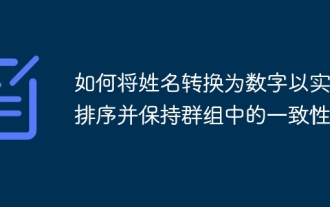
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
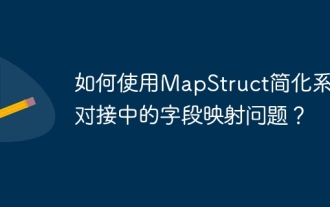
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
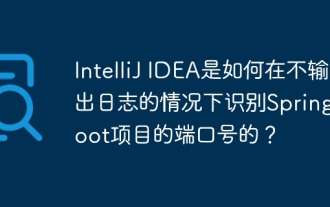
Start Spring using IntelliJIDEAUltimate version...
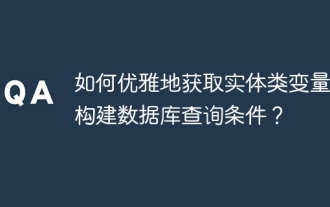
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
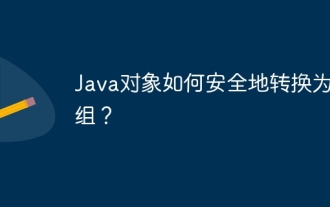
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
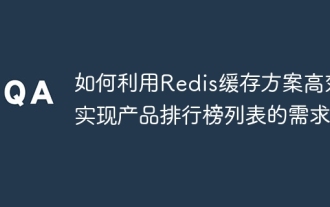
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
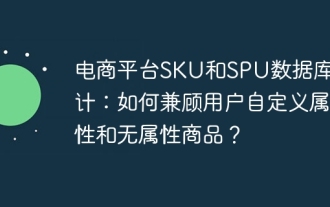
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
