Python None Comparison: When Should I Use 'is' vs. '=='?
Python None Comparison: When to Use "is" vs. ==
When working with the None value in Python, developers often use both "is" and "==" for comparison. Though both approaches are syntactically valid, the recommended choice depends on the intended purpose of the comparison.
Generally, "is" is preferred when checking for object identity, meaning whether two variables refer to the exact same object in memory. In contrast, "==" checks for equality, which can vary based on the object's implementation.
Consider the following custom class that overrides the eq method to define equivalence differently from object identity:
class Negator(object): def __eq__(self,other): return not other thing = Negator() print(thing == None) # True print(thing is None) # False
In this example, "==" returns True because Negator's eq method overrides the default behavior. However, "is" returns False because the two variables do not refer to the same object.
When dealing with custom classes where object identity is not critical, "==" is the appropriate choice for checking equality. For example, if you want to compare the contents of two lists, you would use "==":
lst = [1,2,3] lst == lst[:] # This is True since the lists are "equivalent" lst is lst[:] # This is False since they're actually different objects
In contrast, if you want to specifically check if two variables point to the exact same object in memory, "is" is the preferred comparison operator. This is particularly important when working with mutable objects, such as dictionaries or lists, where changing one instance may affect the other if they are the same object:
a = [1, 2, 3] b = a b.append(4) print(a, b) # Output: [1, 2, 3, 4], [1, 2, 3, 4] c = [1, 2, 3] d = c d is c # True (same object in memory)
The above is the detailed content of Python None Comparison: When Should I Use 'is' vs. '=='?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










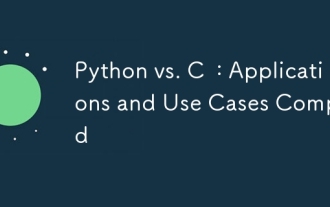
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
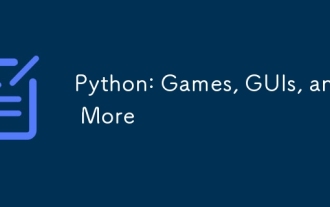
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
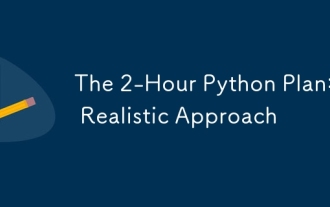
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
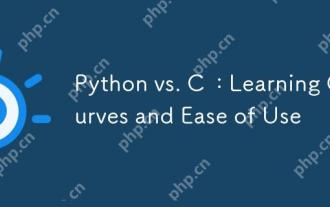
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
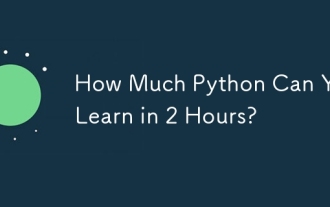
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
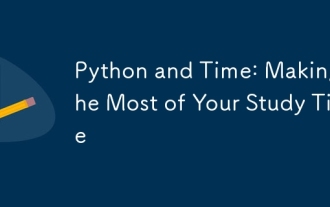
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
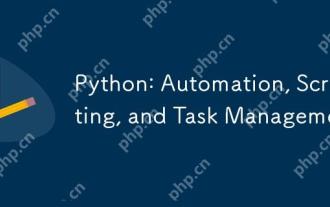
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
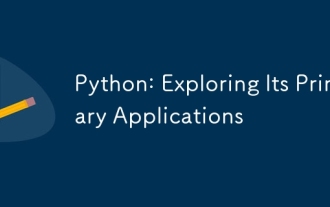
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
