


State Management with useContext and useReducer in React: Building a Global Shopping Cart
Advanced State Management with useContext and useReducer in React: Building a Global Shopping Cart
In the previous article, we introduced the concept of combining useContext and useReducer to manage global state effectively in a React application. We demonstrated this by building a simple to-do list. Now, we’re going to take things up a notch and apply these concepts to a more complex, real-world example—a global shopping cart.
This guide will cover how to manage multiple states and actions, such as adding, updating, and removing items, and calculating totals—all while keeping the application scalable and performant.
In this second part, you’ll learn to:
- Handle more complex states using useReducer.
- Create a flexible context provider to manage state and actions globally.
- Implement advanced reducer functions to perform calculations and handle various types of actions.
- Optimize component performance with memoization for better performance.
Let's dive in!
Project Overview: A Global Shopping Cart
Our shopping cart application will have:
- Product List: A set of items available to add to the cart.
- Cart Functionality: Users can add, update, and remove items in the cart.
- Cart Totals: Calculate and display the total items and the total price.
We’ll start by setting up the context and reducer, then build components to showcase the features.
Setup and Initial Files
To get started, initialize your React project and set up a basic folder structure:
src/ ├── CartContext.js ├── CartProvider.js ├── ProductList.js ├── Cart.js └── App.js
Step 1: Create the Initial State and Reducer
We’ll start with an initial state that represents an empty cart and a set of sample products.
Initial State:
// Initial state structure const initialState = { products: [ { id: 1, name: "Product A", price: 30 }, { id: 2, name: "Product B", price: 20 }, { id: 3, name: "Product C", price: 50 } ], cart: [], totalItems: 0, totalPrice: 0 };
Reducer Function:
We’ll set up a cartReducer function to handle various actions such as adding items, updating item quantities, removing items, and calculating totals.
src/ ├── CartContext.js ├── CartProvider.js ├── ProductList.js ├── Cart.js └── App.js
Explanation
- ADD_TO_CART: Adds an item to the cart, increasing the quantity if it already exists.
- REMOVE_FROM_CART: Removes an item based on its ID.
- UPDATE_QUANTITY: Updates the quantity of an item in the cart.
- CALCULATE_TOTALS: Calculates the total number of items and the total price of the cart.
Step 2: Create Context and Provider
Now, we’ll create a context and provider to pass our state and dispatch function globally. This will allow all components to access the cart state and actions.
CartContext.js
// Initial state structure const initialState = { products: [ { id: 1, name: "Product A", price: 30 }, { id: 2, name: "Product B", price: 20 }, { id: 3, name: "Product C", price: 50 } ], cart: [], totalItems: 0, totalPrice: 0 };
Step 3: Building the Components
With the provider and context set up, we can now create components for the Product List and the Cart.
ProductList Component
The ProductList component will display a list of available products and allow users to add products to the cart.
ProductList.js
function cartReducer(state, action) { switch (action.type) { case "ADD_TO_CART": { const item = state.cart.find(item => item.id === action.payload.id); const updatedCart = item ? state.cart.map(cartItem => cartItem.id === item.id ? { ...cartItem, quantity: cartItem.quantity + 1 } : cartItem ) : [...state.cart, { ...action.payload, quantity: 1 }]; return { ...state, cart: updatedCart }; } case "REMOVE_FROM_CART": { const updatedCart = state.cart.filter(item => item.id !== action.payload); return { ...state, cart: updatedCart }; } case "UPDATE_QUANTITY": { const updatedCart = state.cart.map(item => item.id === action.payload.id ? { ...item, quantity: action.payload.quantity } : item ); return { ...state, cart: updatedCart }; } case "CALCULATE_TOTALS": { const { totalItems, totalPrice } = state.cart.reduce( (totals, item) => { totals.totalItems += item.quantity; totals.totalPrice += item.price * item.quantity; return totals; }, { totalItems: 0, totalPrice: 0 } ); return { ...state, totalItems, totalPrice }; } default: return state; } }
Cart Component
The Cart component displays items in the cart, allows updating quantities, removing items, and shows the total items and price.
Cart.js
import React, { createContext, useReducer } from 'react'; export const CartContext = createContext(); export function CartProvider({ children }) { const [state, dispatch] = useReducer(cartReducer, initialState); return ( <CartContext.Provider value={{ state, dispatch }}> {children} </CartContext.Provider> ); }
Explanation
- handleRemove: Removes an item from the cart.
- handleUpdateQuantity: Updates the quantity of a selected item.
- Total Items and Price: The cart component displays the total items and price based on the calculated values in the state.
Step 4: Wrap the App with the Provider
To ensure all components can access the cart state, wrap the entire app in CartProvider.
App.js
import React, { useContext } from 'react'; import { CartContext } from './CartContext'; function ProductList() { const { state, dispatch } = useContext(CartContext); const handleAddToCart = (product) => { dispatch({ type: "ADD_TO_CART", payload: product }); dispatch({ type: "CALCULATE_TOTALS" }); }; return ( <div> <h2>Products</h2> <ul> {state.products.map(product => ( <li key={product.id}> {product.name} - ${product.price} <button onClick={() => handleAddToCart(product)}>Add to Cart</button> </li> ))} </ul> </div> ); } export default ProductList;
Final Touches: Memoization and Optimization
As your application grows, optimizing performance is essential. Here are a few tips:
- Memoize Components: Use React.memo to prevent unnecessary re-renders of components that depend on state.
- Separate Contexts: Consider separating product and cart contexts if they become too large, allowing more targeted state updates.
Recap and Conclusion
In this advanced guide, we used useContext and useReducer to manage a global shopping cart. Key takeaways include:
- Complex State Management: useReducer simplifies managing complex actions and calculations.
- Global State with useContext: Makes state accessible across the component tree.
- Scalable Patterns: Separating state and actions in contexts allows for cleaner, more modular code.
Try applying this approach to your projects, and see how it improves the scalability and performance of your applications. Happy coding! ?
The above is the detailed content of State Management with useContext and useReducer in React: Building a Global Shopping Cart. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
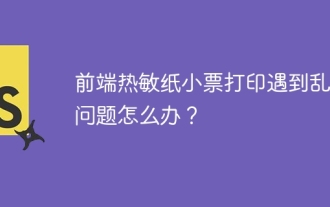
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
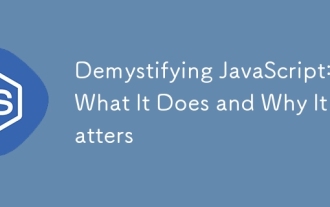
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
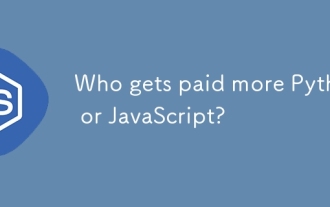
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
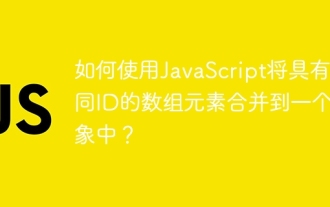
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
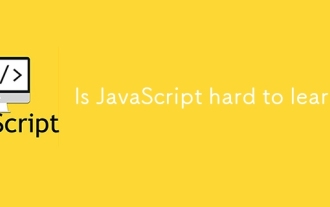
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
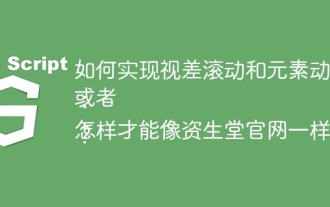
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
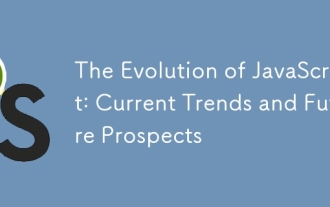
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
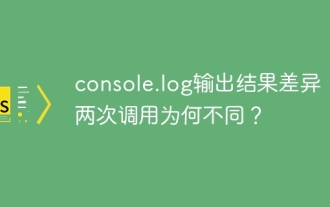
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
