How Can I Handle Errors in Goroutines When Using Channels?
Passing Errors in Goroutines Using Channels
In Go, functions typically return a value and an optional error, as demonstrated in the provided createHashedPassword function. When executing this function in a goroutine, a common approach is to communicate the results through a channel. However, handling errors within this setup requires special consideration.
To effectively handle errors in goroutines, it's recommended to encapsulate the output values, including potential errors, into a custom struct. By passing this struct over a single channel, you can return both the result and any associated errors effortlessly.
For example, you could create a Result struct with two fields: Message for the expected output and Error for any encountered errors:
type Result struct { Message string Error error }
Next, initialize a channel specifically for transmitting Result structs:
ch := make(chan Result)
Now, within your goroutine, execute the createHashedPassword function and assign the result to a Result variable:
go func() { result := Result{ Message: createHashedPassword(), Error: err, // Any potential error encountered during execution } ch <- result }()
On the receiving end, you can retrieve the result and check for any errors:
select { case result := <-ch: if result.Error != nil { // Handle the error } // Do something with result.Message }
The above is the detailed content of How Can I Handle Errors in Goroutines When Using Channels?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


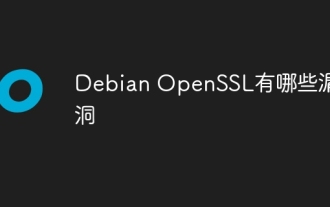
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
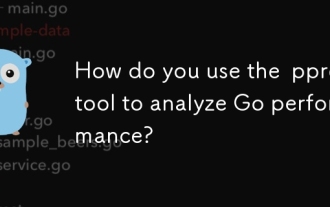
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
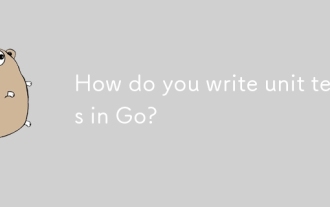
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
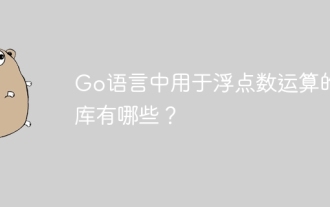
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
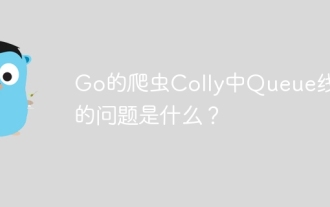
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
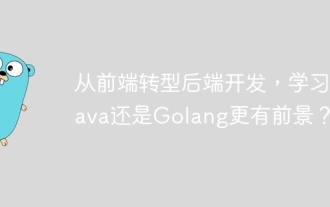
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
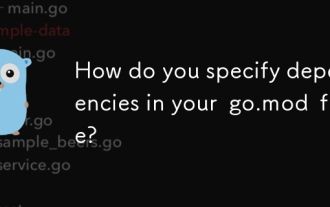
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
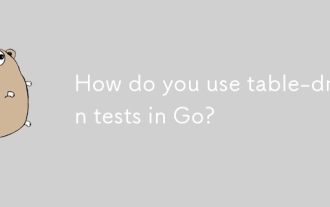
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
