


How to Execute Multiple SQL Queries in a Single Result Set with PHP/MySQL?
Executing Multiple Queries in PHP/MySQL
Question:
How can I combine multiple SQL queries into a single result set in PHP using MySQL?
Answer:
To execute multiple concatenated queries in PHP using MySQL, follow these steps:
-
Create the SQL Statement:
Concatenate the individual SQL queries into a single string, separated by semicolons (;). Ensure there are two semicolons at the end of each query except the last one. -
Connect to Database:
Use the mysqli_connect function to establish a database connection. -
Prepare Query:
Use the mysqli_prepare function to prepare the multiple-query statement.
$query = "SQL STATEMENT 1;;SQL STATEMENT 2;;SQL STATEMENT 3";
-
Execute Queries:
Use the mysqli_multi_query function to execute the prepared statement.
if (mysqli_multi_query($link, $query)) { do { // Store and process results } while (mysqli_next_result($link)); }
-
Fetch Results:
After executing the queries, use the mysqli_fetch_array to retrieve results from each query.
Example:
$link = mysqli_connect("server", "user", "password", "database"); $query = "CREATE VIEW current_rankings AS SELECT * FROM main_table WHERE date = X;; CREATE VIEW previous_rankings AS SELECT rank FROM main_table WHERE date = date_sub('X', INTERVAL 1 MONTH);; CREATE VIEW final_output AS SELECT current_rankings.player, current_rankings.rank as current_rank LEFT JOIN previous_rankings.rank as prev_rank ON (current_rankings.player = previous_rankings.player);; SELECT *, @rank_change = prev_rank - current_rank as rank_change from final_output"; if (mysqli_multi_query($link, $query)) { do { if ($result = mysqli_store_result($link)) { while ($row = mysqli_fetch_array($result)) { // Process results } mysqli_free_result($result); } } while (mysqli_next_result($link)); }
Alternative Approach:
Alternatively, you can execute each query separately and process the results one by one. This approach is recommended if the queries are not interdependent.
$query1 = "Create temporary table A select c1 from t1"; $result1 = mysqli_query($link, $query1); $query2 = "select c1 from A"; $result2 = mysqli_query($link, $query2); while($row = mysqli_fetch_array($result2)) { // Process results }
The above is the detailed content of How to Execute Multiple SQL Queries in a Single Result Set with PHP/MySQL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










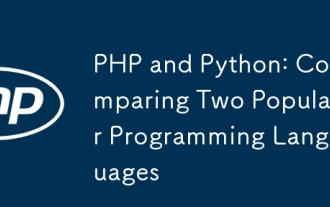
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
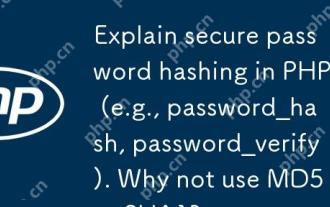
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
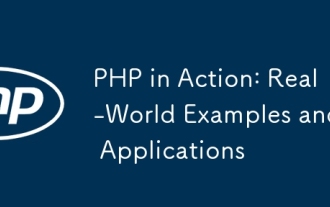
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
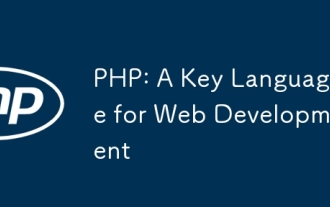
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
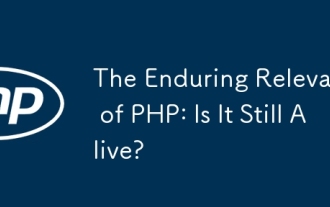
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
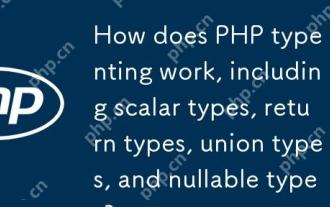
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
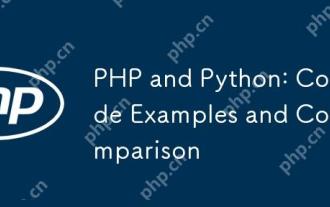
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
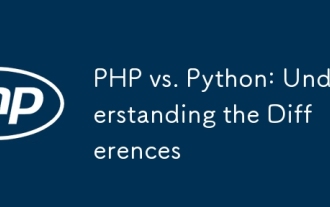
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
