How to Pass $_GET Variables to a Bootstrap Modal?
How to Pass $_GET Variables from a Link to a Bootstrap Modal
Passing $_GET variables from a link to a Bootstrap modal is essential for modifying and displaying specific data within a modal. Here are several solutions to achieve this:
Simple Solution
-
Modal Call Button:
<td data-placement="top" data-toggle="tooltip" title="Show"> <a class="btn btn-primary btn-xs" data-toggle="modal" data-target="#editBox" href="file.php?id=<;?php echo $obj->id; ?>""> <span class="glyphicon glyphicon-pencil"></span> </a> </td>
Copy after login -
Modal HTML:
<div class="modal fade">
Copy after loginCopy after loginCopy after login -
file.php
$Id = $_GET["id"]; // Escape the string if needed // Run query to fetch records against $Id // Show records in modal body
Copy after login
Alternate Solution with Ajax and Bootstrap Modal Event Listener
-
Modal Call Button:
<td data-placement="top" data-toggle="tooltip" title="Show"> <a class="btn btn-primary btn-xs" data-toggle="modal" data-target="#editBox" data-id="<php echo $obj->id; ?>"> <span class="glyphicon glyphicon-pencil"></span> </a> </td>
Copy after login -
Modal HTML:
<div class="modal fade">
Copy after loginCopy after loginCopy after login -
Script:
$( document ).ready(function() { $('#myModal').on('show.bs.modal', function (e) { var id = $(e.relatedTarget).data('id'); $.ajax({ type : 'post', url : 'file.php', // Fetches records data : 'post_id='+ id, success : function(data) { $('.form-data').html(data); // Shows fetched data in modal body } }); }); });
Copy after login -
file.php
if ($_POST['id']) { $id = $_POST['id']; // Run query to fetch records // Echo the data to be displayed in the modal }
Copy after loginCopy after login
Alternate Solution with Ajax and jQuery Click Function
-
Modal Call Button:
<td data-placement="top" data-toggle="tooltip" title="Show"> <a class="btn btn-primary btn-xs open-modal" href="">
Copy after login -
Modal HTML:
<div class="modal fade">
Copy after loginCopy after loginCopy after login -
Script:
$( document ).ready(function() { $('.open-modal').click(function(){ var id = $(this).attr('id'); $.ajax({ type : 'post', url : 'file.php', // Fetches records data : 'post_id='+ id, success : function(data) { $('#editBox').show('show'); $('.form-data').html(data); } }); }); });
Copy after login -
file.php
if ($_POST['id']) { $id = $_POST['id']; // Run query to fetch records // Echo the data to be displayed in the modal }
Copy after loginCopy after login
Pass On-Page Information to Modal
-
Modal Call Button:
<td data-placement="top" data-toggle="tooltip" title="Show"> <a data-book-id="<php echo $obj->id; ?>" data-name="<php echo $obj->name; ?>" data-email="<php echo $obj->email; ?>" class="btn btn-primary btn-xs" data-toggle="modal" data-target="#editBox"> <span class="glyphicon glyphicon-pencil"></span> </a> </td>
Copy after login -
Modal Event:
$(document).ready(function(){ $('#editBox').on('show.bs.modal', function (e) { var bookid = $(e.relatedTarget).data('book-id'); var name = $(e.relatedTarget).data('name'); var email = $(e.relatedTarget).data('email'); // Can pass as many on-page values to modal }); });
Copy after login
By implementing one of these solutions, you can effectively pass $_GET variables from a link to a Bootstrap modal, allowing you to load and manipulate data dynamically within a modal window.
The above is the detailed content of How to Pass $_GET Variables to a Bootstrap Modal?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


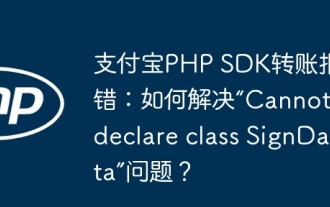
Alipay PHP...
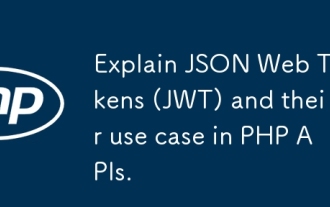
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
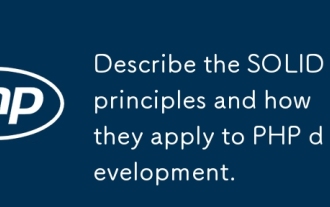
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
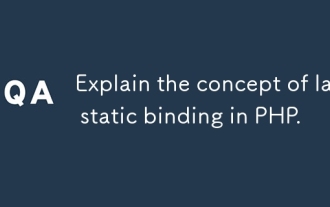
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
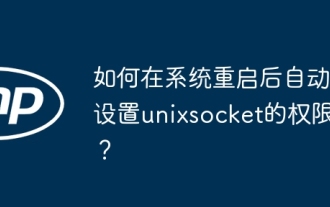
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
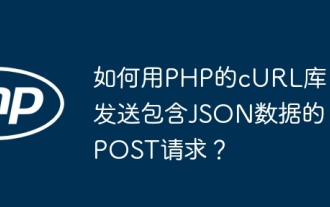
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
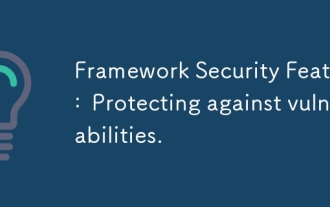
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
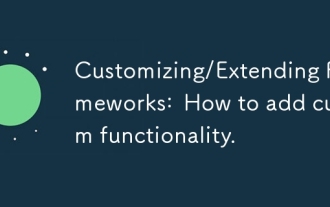
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
