


Why does json.Marshal with json.RawMessage return a Base64 encoded string?
Marshalling json.RawMessage Returns Base64 Encoded String
When calling json.Marshal with a json.RawMessage value, the result is unexpected. Instead of the desired JSON string, a base64 encoded string is returned.
package main import ( "encoding/json" "fmt" ) func main() { raw := json.RawMessage(`{"foo":"bar"}`) j, err := json.Marshal(raw) if err != nil { panic(err) } fmt.Println(string(j)) // Output: "eyJmb28iOiJiYXIifQ==" }
The issue lies in the usage of json.RawMessage in json.Marshal. The json.RawMessage type, designed to store raw JSON data without decoding it, has a MarshalJSON method that simply returns the byte slice.
func (m *RawMessage) MarshalJSON() ([]byte, error) { return *m, nil }
However, for json.Marshal to function correctly with json.RawMessage, the value passed must be a pointer to the json.RawMessage instance.
j, err := json.Marshal(&raw)
By passing a pointer to json.RawMessage, the MarshalJSON method is invoked on the pointer, ensuring that the byte slice is returned without base64 encoding.
package main import ( "encoding/json" "fmt" ) func main() { raw := json.RawMessage(`{"foo":"bar"}`) j, err := json.Marshal(&raw) if err != nil { panic(err) } fmt.Println(string(j)) // Output: "{"foo":"bar"}" }
The above is the detailed content of Why does json.Marshal with json.RawMessage return a Base64 encoded string?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




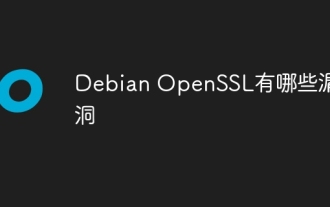
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
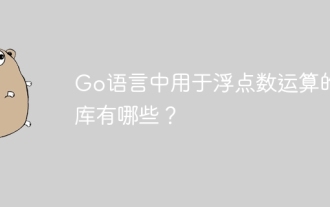
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
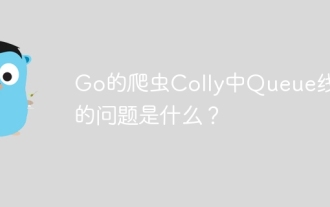
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
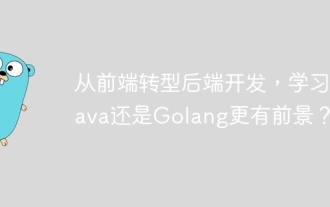
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
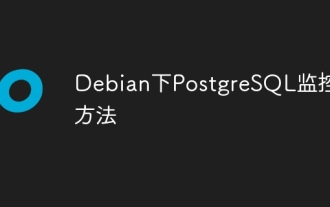
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
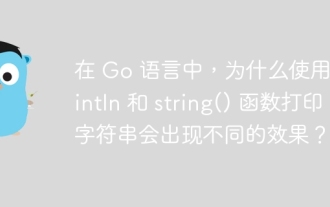
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
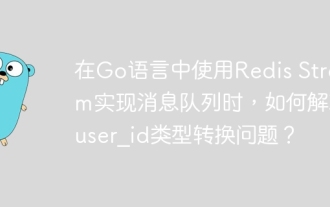
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
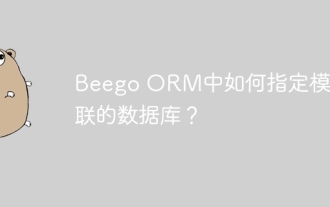
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
