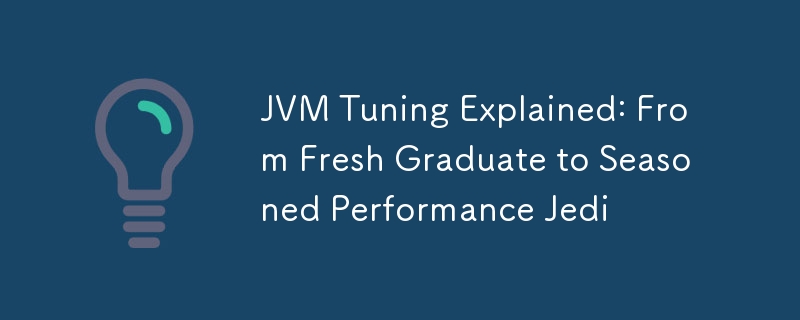
Ah, the JVM (Java Virtual Machine). To some, it's a mystical black box. To others, it's a battleground where wars are waged over milliseconds and memory allocation. Regardless of your background, understanding how to tune the JVM is akin to having the keys to the kingdom of Java performance. This article takes you on an epic journey from the basics to expert-level insights on JVM tuning, so grab your cup of coffee, or two — this is going to be a wild ride.
Chapter 1: What Is the JVM and Why Do We Tune It?
Before tuning, it’s crucial to know what exactly we're tuning. The JVM is essentially the engine that powers Java applications. It manages program execution and is responsible for converting your bytecode into machine code that your computer can execute.
Why Tune the JVM?
-
Performance Issues: Slow response times? Lagging? Out of memory errors? Welcome to JVM tuning!
-
Resource Management: Make sure your application isn't a memory hog.
-
Scalability: Ensure your application can handle an increasing number of users or data.
When Should You Tune the JVM?
-
Application Slowness: When your app feels like it’s running through molasses.
-
High Latency: When response times creep up, and users start refreshing their pages in anger.
-
Out of Memory (OOM) Errors: The dreaded java.lang.OutOfMemoryError.
-
CPU Bottlenecks: When your app starts to resemble a hungry monster gobbling CPU cycles.
-
GC (Garbage Collection) Stalls: Pauses that make your application stop to ponder life’s mysteries.
Chapter 2: Anatomy of JVM Memory — Know Your Heap and Friends
JVM Memory Structure Overview
The JVM memory is divided into different regions:
-
Heap Memory: Where Java objects live. Divided into:
- Young Generation (Eden Survivor spaces)
- Old Generation (Tenured space)
-
Non-Heap Memory: Includes:
-
Metaspace (Post-Java 8, previously PermGen)
- Code Cache
-
Stack Memory: For method call execution and local variable storage.
-
Direct Memory: Used for NIO operations.
// Quick visualization of JVM memory structure
/*
----------------------------
| Stack Memory |
----------------------------
| Non-Heap Memory |
| --------------------- |
| | Metaspace | |
| | Code Cache | |
| --------------------- |
| |
----------------------------
| Heap Memory |
| --------------------- |
| | Young Gen | |
| | | Eden | | |
| | |Survivor Space | | |
| --------------------- |
| | Old Gen | |
| --------------------- |
----------------------------
*/
Copy after login
Copy after login
Copy after login
Chapter 3: The JVM Garbage Collection (GC) Dance
The JVM's garbage collectors are like your app’s janitors, tidying up memory by collecting and removing unneeded objects.
Types of Garbage Collectors:
-
Serial GC: Single-threaded, simple, and great for single-threaded apps or smaller heaps. Use case: Embedded systems.
-
Parallel GC (Throughput Collector): Multi-threaded, designed for high throughput. Use case: Apps where response time isn’t a big deal.
-
G1 (Garbage-First) GC: Splits the heap into regions, prioritizes garbage collection to minimize pauses. Use case: General-purpose, low-latency applications.
-
ZGC: Ultra-low-latency, designed for heaps up to terabytes. Use case: When you’re running apps that need to respond quickly and have massive data.
-
Shenandoah GC: Another low-latency collector with concurrent compaction. Use case: Similar to ZGC, great for real-time applications.
Tuning Tips:
-
Understand Your GC Logs: Turn on XX: PrintGCDetails to analyze garbage collection logs.
-
Experiment with Flags:
// Quick visualization of JVM memory structure
/*
----------------------------
| Stack Memory |
----------------------------
| Non-Heap Memory |
| --------------------- |
| | Metaspace | |
| | Code Cache | |
| --------------------- |
| |
----------------------------
| Heap Memory |
| --------------------- |
| | Young Gen | |
| | | Eden | | |
| | |Survivor Space | | |
| --------------------- |
| | Old Gen | |
| --------------------- |
----------------------------
*/
Copy after login
Copy after login
Copy after login
Chapter 4: JVM Parameters — A Developer’s Arsenal
Common JVM Flags:
Flag |
Description |
-Xms |
Initial heap size |
-Xmx |
Maximum heap size |
-XX:NewRatio= |
Ratio between young and old generation |
-XX:SurvivorRatio= |
Size ratio of the survivor spaces to Eden |
-XX: UseG1GC |
Use G1 Garbage Collector |
-XX: PrintGCDetails |
Prints detailed GC logs |
-XX: HeapDumpOnOutOfMemoryError |
Dumps heap when OOM error occurs |
Flag |
Description |
-Xms |
Initial heap size |
-Xmx |
Maximum heap size |
-XX:NewRatio= |
Ratio between young and old generation |
-XX:SurvivorRatio= |
Size ratio of the survivor spaces to Eden |
-XX: UseG1GC |
Use G1 Garbage Collector |
-XX: PrintGCDetails |
Prints detailed GC logs |
-XX: HeapDumpOnOutOfMemoryError |
Dumps heap when OOM error occurs |
Setting Heap Size:
For optimal heap size tuning:
-
Initial Heap (Xms) and Max Heap (Xmx): Set both to avoid runtime resizing. Keep these equal for stable performance.
-
Rule of Thumb: Xms should be around 1/4 of your system RAM, and Xmx should never exceed 50% of it.
GC Tuning Parameters:
For G1GC:
// Quick visualization of JVM memory structure
/*
----------------------------
| Stack Memory |
----------------------------
| Non-Heap Memory |
| --------------------- |
| | Metaspace | |
| | Code Cache | |
| --------------------- |
| |
----------------------------
| Heap Memory |
| --------------------- |
| | Young Gen | |
| | | Eden | | |
| | |Survivor Space | | |
| --------------------- |
| | Old Gen | |
| --------------------- |
----------------------------
*/
Copy after login
Copy after login
Copy after login
-
MaxGCPauseMillis: Target pause time for GC.
-
InitiatingHeapOccupancyPercent: Percentage that triggers a GC cycle.
Monitoring with JVisualVM and JConsole
To visualize memory usage:
-
JVisualVM: Perfect for monitoring heap size, GC activity, and thread states.
-
JConsole: Lightweight, great for quick peeks at memory and thread status.
Chapter 5: Practical Tuning Scenarios
Scenario 1: High Latency Spikes
Symptoms: Latency spikes during peak traffic.
Solution: Use G1GC with -XX:MaxGCPauseMillis tuned to a reasonable target (e.g., 200 ms).
Scenario 2: Out of Memory (OOM) Errors
Symptoms: java.lang.OutOfMemoryError after sustained load.
Solution:
-
Increase Heap Size: Xmx4g
-
Enable Heap Dump: XX: HeapDumpOnOutOfMemoryError
Scenario 3: CPU Thrashing Due to GC
Symptoms: High CPU usage during GC cycles.
Solution: Tune GC threads with -XX:ParallelGCThreads= and use a low-latency GC like ZGC.
Chapter 6: JVM Tuning for Specific Applications
Tuning for Microservices:
-
Lightweight GCs like ZGC or Shenandoah for fast response times.
- Optimize startup time with Xshare:on for class data sharing.
- Monitor with tools like Prometheus Grafana for detailed insights.
Tuning for High-Traffic Web Applications:
-
Load Test First: Use tools like Apache JMeter to simulate traffic.
- Implement load balancers and distribute memory tuning across nodes.
Chapter 7: JVM Tuning Mistakes to Avoid
-
Over-tuning: Adding too many GC flags without proper monitoring can backfire.
-
Not Monitoring: Always monitor post-tuning. Use GC Viewer or GCEasy for insights.
-
Ignoring Non-Heap Memory: Metaspace can lead to issues if not sized properly (XX:MaxMetaspaceSize=256m).
Chapter 8: Beyond JVM Tuning — Profiling Your Application
Tuning the JVM is great, but don't forget:
-
Code Profiling: Use tools like YourKit or VisualVM to find memory leaks and CPU hogs.
-
Optimize Database Calls: Unoptimized queries can bottleneck your app before JVM tuning makes any difference.
Conclusion
JVM tuning isn’t a one-size-fits-all approach. It requires careful analysis, continuous testing, and monitoring. With the tips outlined here, you’re well-equipped to tune the JVM to transform your Java application from a sluggish tortoise into a lightning-fast hare. Now go forth and tune, JVM warrior!
Further Reading and Resources
- "Java Performance: The Definitive Guide" by Scott Oaks
BUY || PDF
- JVM Documentation and Tuning Guide (Oracle)
-
GC Viewer and Eclipse MAT for memory analysis.
Remember: JVM tuning is part science, part art, and a lot of patience. Happy tuning!
The above is the detailed content of JVM Tuning Explained: From Fresh Graduate to Seasoned Performance Jedi. For more information, please follow other related articles on the PHP Chinese website!