How to Safely Remove Entries from a Concurrent Map While Iterating?
Iterating Over and Removing from a Concurrent Map
When modifying a map during iteration, it's crucial to avoid ConcurrentModificationException errors. The classic approach of using for (Object key : map.keySet()) fails because map modifications can occur during the loop.
A common workaround is to copy the key set into a new collection, as seen in for (Object key : new ArrayList
A better solution involves using an iterator to remove map entries. Here's an example:
Map<String, String> map = new HashMap<>(); map.put("test", "test123"); map.put("test2", "test456"); for(Iterator<Map.Entry<String, String>> it = map.entrySet().iterator(); it.hasNext(); ) { Map.Entry<String, String> entry = it.next(); if(entry.getKey().equals("test")) { it.remove(); } }
This code uses an iterator to safely remove entries from the map. The iterator's remove() method removes the current entry, allowing for safe and efficient map modifications during iteration.
The above is the detailed content of How to Safely Remove Entries from a Concurrent Map While Iterating?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










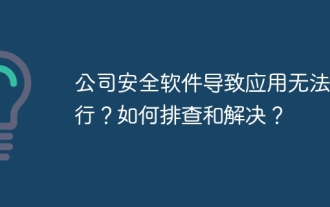
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
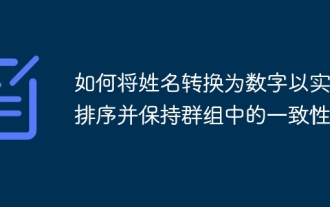
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
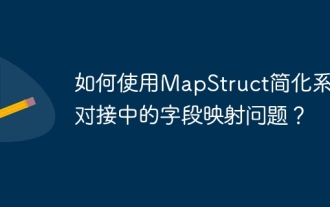
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
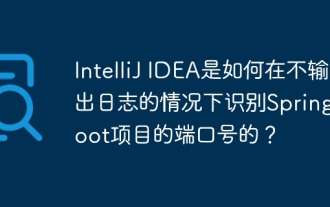
Start Spring using IntelliJIDEAUltimate version...
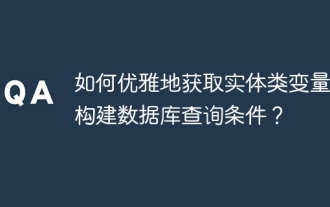
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
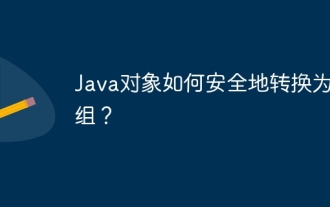
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
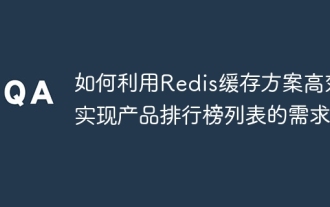
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
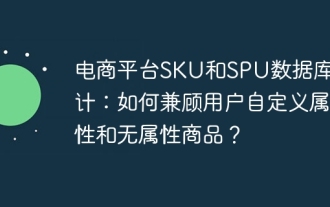
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
