How to Achieve Multiple Assignments from Arrays in Go?
Nov 12, 2024 am 11:35 AMMultiple Assignments from Arrays in Go
In Python, unpacking from arrays can be done elegantly with assignments like:
a, b = "foo;bar".split(";")
Go does not support such general packing/unpacking. However, there are several ways to achieve multiple assignments.
Custom Functions:
One approach is to create a custom function that returns multiple values, like:
func splitLink(s, sep string) (string, string) { x := strings.Split(s, sep) return x[0], x[1] }
You can then assign directly from the function call:
name, link := splitLink("foo\thttps://bar", "\t")
Variadic Pointer Arguments:
Another option is to use variadic pointer arguments:
func unpack(s []string, vars... *string) { for i, str := range s { *vars[i] = str } }
This allows you to assign values to multiple variables:
var name, link string unpack(strings.Split("foo\thttps://bar", "\t"), &name, &link)
Choice of Approach:
The custom function approach may be more readable for common scenarios where you want to split and assign only two variables. For more complex or variable-sized array scenarios, the variadic pointer arguments approach may be more flexible.
The above is the detailed content of How to Achieve Multiple Assignments from Arrays in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
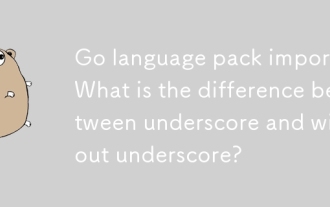
Go language pack import: What is the difference between underscore and without underscore?
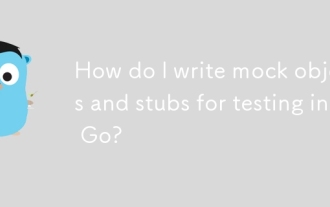
How do I write mock objects and stubs for testing in Go?

How to implement short-term information transfer between pages in the Beego framework?
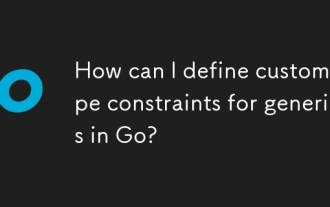
How can I define custom type constraints for generics in Go?
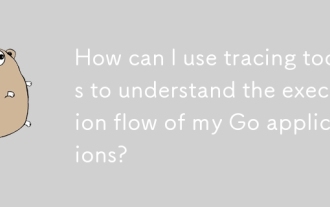
How can I use tracing tools to understand the execution flow of my Go applications?
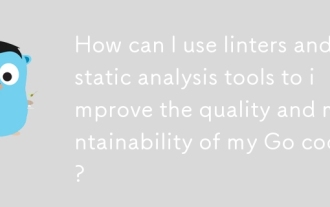
How can I use linters and static analysis tools to improve the quality and maintainability of my Go code?
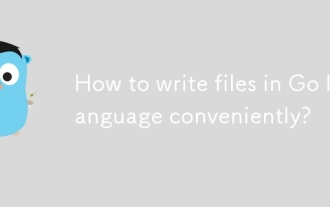
How to write files in Go language conveniently?
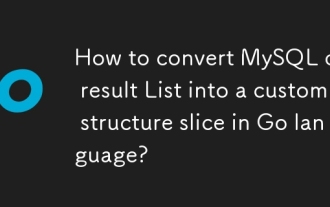
How to convert MySQL query result List into a custom structure slice in Go language?
