Can Go Channels Mimic Python Generators?
Python-Style Generators in Go
In Go, goroutines can be utilized like Python generators, as exemplified in Question 66 of the Tour of Go. The provided code rewrites Question 66 in a simplified manner:
package main import "fmt" func fibonacci(c chan int) { x, y := 1, 1 for { c <- x x, y = y, x + y } } func main() { c := make(chan int) go fibonacci(c) for i := 0; i < 10; i++ { fmt.Println(<-c) } }
Analysis
- Increasing the channel's buffer size will result in a performance boost due to reduced context switching. However, this comes at the cost of increased memory usage.
- Memory leakage occurs when goroutines are not garbage-collected. In the provided code, the fibonacci goroutine persists indefinitely, preventing channel c from being garbage-collected.
Alternative Approach
To address these issues, consider the following code:
package main import "fmt" func fib(n int) chan int { c := make(chan int) go func() { x, y := 0, 1 for i := 0; i <= n; i++ { c <- x x, y = y, x+y } close(c) }() return c } func main() { for i := range fib(10) { fmt.Println(i) } }
In this example:
- The fib function returns a channel, allowing the caller to iteratively access Fibonacci numbers up to a specified limit.
- The fibonacci goroutine closes the channel when it reaches the end, ensuring that memory leakage does not occur.
The above is the detailed content of Can Go Channels Mimic Python Generators?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










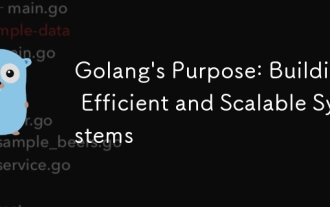
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
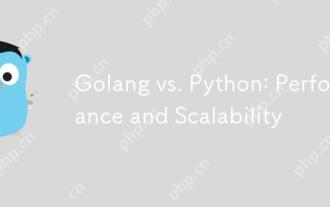
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
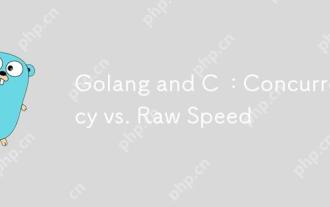
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
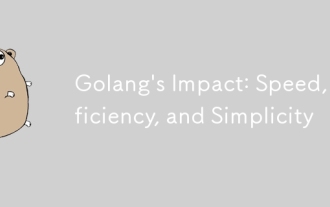
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
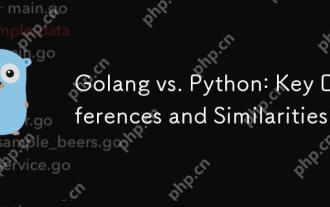
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
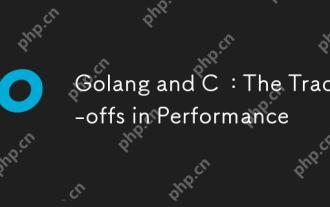
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
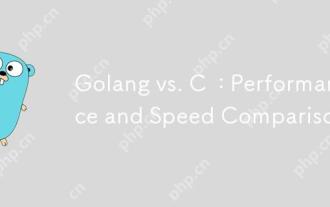
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
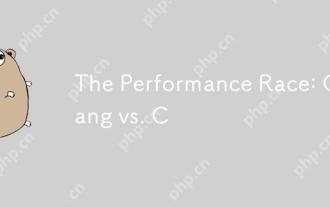
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
