


How do I efficiently merge multiple dataframes based on a common date column?
Merging Multiple Dataframes Based on Date
You have multiple dataframes with a common date column but varying numbers of rows and columns. The goal is to merge these dataframes to obtain rows where each date is common to all dataframes.
Inefficient Recursion Approach
Your attempt to use a recursion function to merge dataframes is flawed. The function enters an infinite loop because it continuously calls itself with the same inputs. This approach is inefficient and prone to errors.
Optimized Solution Using reduce
A more efficient method for merging multiple dataframes is to use the reduce function from the functools module. This function reduces a list of dataframes into a single dataframe by repeatedly applying a specified merge operation to adjacent pairs of dataframes.
The following code snippet demonstrates this approach:
1 2 3 4 5 6 |
|
In this code, the reduce function reduces the dfs list into a single dataframe by iteratively merging adjacent pairs of dataframes. The on='date' parameter specifies that the merge should be performed based on the date column. The how='outer' parameter ensures that all rows from both dataframes are included in the merged result, even if they do not share the same date.
Advantages of reduce Function
Using the reduce function offers several advantages:
- Simplicity: The code is concise and easy to understand.
- No Nesting: Unlike your recursion approach, there is no nesting of merge operations, eliminating the risk of infinite loops.
- Extensibility: You can add or remove dataframes from the dfs list to change the merge operation dynamically.
Example
Using the provided dataframes df1, df2, and df3, you would obtain the following merged dataframe:
1 2 |
|
This dataframe contains only rows with a date that is common to all three input dataframes.
The above is the detailed content of How do I efficiently merge multiple dataframes based on a common date column?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










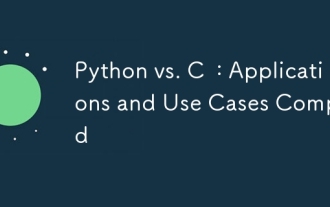
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
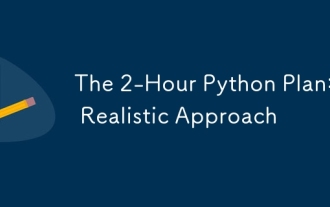
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
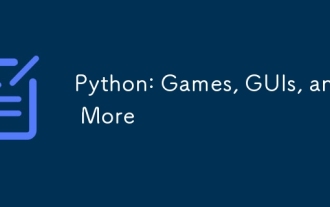
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
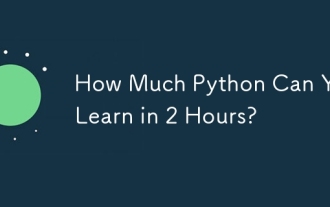
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
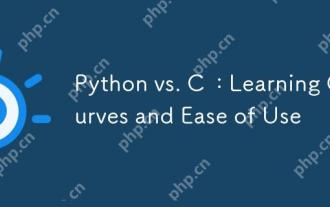
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
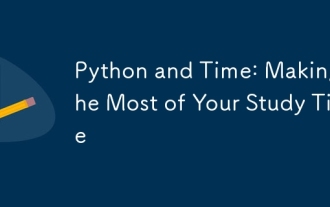
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
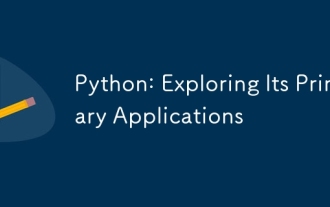
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
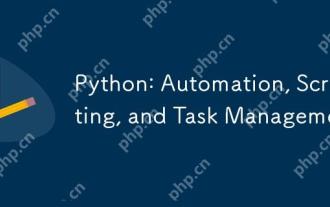
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
