How to Validate Uploaded File Types in PHP with `in_array()`?
Uploading Specified File Types with PHP
In web applications, allowing users to upload files requires careful consideration of security and content validation. When you want to restrict uploads to specific file types, PHP provides a solution through the in_array() function.
Problem:
You wish to create an if statement in PHP to validate uploaded files and allow only files of the following types: jpg, gif, and pdf. The code below requires the appropriate structuring of the if statement.
$file_type = $_FILES['foreign_character_upload']['type']; //returns the mimetype if(/*$file_type is anything other than jpg, gif, or pdf*/) { $error_message = 'Only jpg, gif, and pdf files are allowed.'; $error = 'yes'; }
Solution:
To ensure files uploaded conform to your specifications, create an array of allowed file types and utilize in_array() to determine whether the uploaded file's mimetype is included in the array.
$file_type = $_FILES['foreign_character_upload']['type']; //returns the mimetype $allowed = array("image/jpeg", "image/gif", "application/pdf"); if(!in_array($file_type, $allowed)) { $error_message = 'Only jpg, gif, and pdf files are allowed.'; $error = 'yes'; }
By comparing the file type against the predefined list of allowed types, this revised if statement effectively prevents uploads of non-compliant files.
The above is the detailed content of How to Validate Uploaded File Types in PHP with `in_array()`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


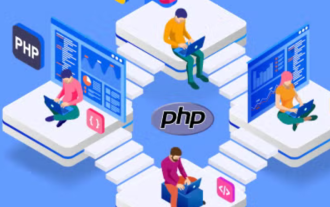
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
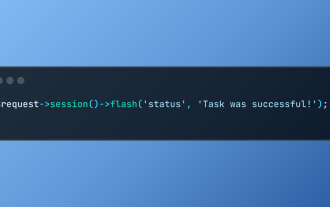
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
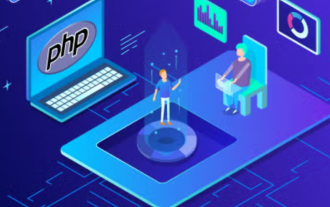
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
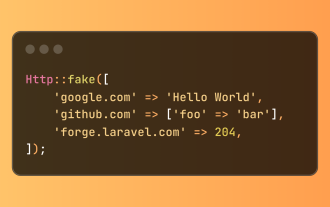
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
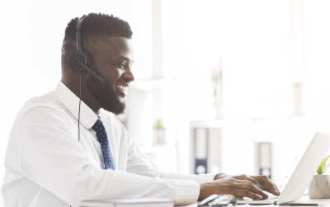
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
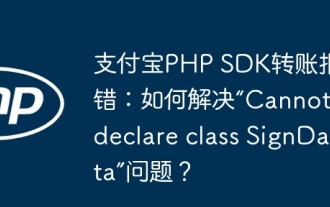
Alipay PHP...
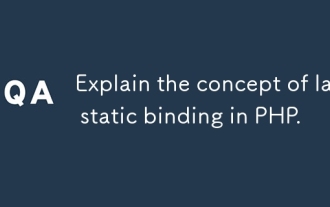
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
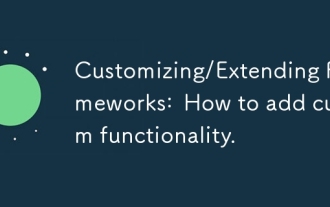
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
