Node.js Starter Project dengan GraphQL, Redis, JWT, dan Sequelize
This template provides a Node.js Starter Project preconfigured with GraphQL for API, Redis for caching and temporary data storage, JWT for authentication and authorization, as well as Sequelize for ORMs connected to relational databases such as PostgreSQL or MySQL. The project has a modular structure that allows you to instantly develop modern web applications with integrated and scalable features.
? Project Overview
This project is designed to make it easier to develop backend applications with a GraphQL API that uses Redis for data caching and JWT to secure the API. Sequelize is used as an ORM to facilitate interaction with relational databases. In addition, there is middleware that makes it easier to handle authentication, validation and logging.
Key Features
- GraphQL API for more flexible and efficient querying and data mutation
- JWT Authentication for secure token-based authentication
- Redis for data caching and improving application performance
- Sequelize ORM for relational database management
- Middleware for centralized authorization and request handling
- Modular and well-structured for scalability and easier maintenance
?️ Technology Used
- Node.js: Platform for building server-side applications with JavaScript. Learn more
- GraphQL: Query language for APIs that enables efficient and flexible data retrieval. Learn more
- Redis: Temporary data storage (in-memory) which is often used for caching and message brokering. Learn more
- JWT: Secure and simple token-based authentication technology. Learn more
- Sequelize: ORM for Node.js that supports PostgreSQL, MySQL, and other relational databases. Learn more
? Steps to Set Up and Run a Project
1. Clone Repository
First of all, clone this template repository to your local machine:
git clone https://gitlab.com/dioarafi1/graphify-api.git cd graphify-api
If you are starting from scratch, initialize the new project with:
git clone https://gitlab.com/dioarafi1/graphify-api.git cd graphify-api
2. Installation of Dependencies
After cloning the repository or creating a new project, run the command to install the required dependencies:
mkdir blog-api cd blog-api npm init -y
This will install all the dependencies listed in the package.json file.
3. Environment Configuration
Create an .env file in the project root directory and add the following configurations for Redis, JWT, and Database:
yarn install
Change user, password, and mydatabase according to your database configuration.
4. Preparing the Database with Sequelize
If you don't have a database configured, run the command to initialize Sequelize and create the model:
DATABASE_URL="postgresql://user:password@localhost:5432/mydatabase" JWT_SECRET="your_jwt_secret_key" REDIS_HOST="localhost" REDIS_PORT="6379"
This will create a config, models, and migrations directory structure within your project. Next, create the necessary models for the application such as User and Post, and perform migrations to create tables in the database.
yarn sequelize init
Make sure the database is running (for example using PostgreSQL or MySQL).
5. Setting Up GraphQL Server
Install dependencies for Apollo Server and GraphQL:
yarn sequelize db:migrate
After that, create a GraphQL server configuration file, schema, and resolvers. You can configure the GraphQL server in the following way:
src/server.ts
yarn add apollo-server graphql
src/graphql/schema.ts
Define GraphQL schema for queries and mutations:
import { ApolloServer } from 'apollo-server-express'; import express from 'express'; import { typeDefs, resolvers } from './graphql'; import { authenticateJWT } from './middlewares/auth'; import { sequelize } from './config/database'; const app = express(); // Gunakan middleware JWT app.use(authenticateJWT); // Inisialisasi Apollo Server const server = new ApolloServer({ typeDefs, resolvers, context: ({ req }) => ({ user: req.user }), }); server.applyMiddleware({ app }); const PORT = process.env.PORT || 4000; app.listen(PORT, async () => { console.log(`Server running at http://localhost:${PORT}${server.graphqlPath}`); await sequelize.authenticate(); console.log('Database connected'); });
src/graphql/resolvers.ts
Implement resolvers for queries and mutations:
import { gql } from 'apollo-server-express'; export const typeDefs = gql` type User { id: ID! username: String! } type Post { id: ID! title: String! content: String! user: User! } type Query { posts: [Post] post(id: ID!): Post users: [User] } type Mutation { createPost(title: String!, content: String!): Post register(username: String!, password: String!): User login(username: String!, password: String!): String # JWT token } `;
6. Running Server for Development
To run the server in a development environment with hot-reload, use the following command:
import { Post, User } from '../models'; import jwt from 'jsonwebtoken'; import bcrypt from 'bcryptjs'; export const resolvers = { Query: { posts: () => Post.findAll(), post: (_, { id }) => Post.findByPk(id), users: () => User.findAll(), }, Mutation: { createPost: async (_, { title, content }, { user }) => { if (!user) throw new Error('Authentication required'); const post = await Post.create({ title, content, userId: user.id }); return post; }, register: async (_, { username, password }) => { const hashedPassword = await bcrypt.hash(password, 10); const user = await User.create({ username, password: hashedPassword }); return user; }, login: async (_, { username, password }) => { const user = await User.findOne({ where: { username } }); if (!user) throw new Error('User not found'); const match = await bcrypt.compare(password, user.password); if (!match) throw new Error('Invalid password'); const token = jwt.sign({ userId: user.id }, process.env.JWT_SECRET!, { expiresIn: '1h' }); return token; }, }, };
The server will run at http://localhost:4000, and you can access the GraphQL Playground to test API queries and mutations.
? Project Directory Structure
This project directory structure is designed to separate the different parts of the application to make it more modular and easy to maintain:
yarn dev
? Deployments
To prepare the project for production, use the following command to build TypeScript into JavaScript:
/myapp ├── src │ ├── middlewares # Berisi middleware untuk otentikasi (JWT), caching (Redis), dan validasi │ ├── routes # Definisi endpoint API dan resolver GraphQL │ ├── services # Logika bisnis utama dan pengolahan data │ ├── app.ts # File utama untuk inisialisasi aplikasi dan middleware │ ├── graphql # Menyimpan konfigurasi GraphQL, schema, dan resolvers │ ├── models # Model Sequelize untuk mengelola database relasional │ ├── config # File konfigurasi global untuk Redis, JWT, database, dll │ ├── index.ts # Entry point aplikasi, menginisialisasi server dan middleware │ ├── resolvers # Berisi resolver GraphQL untuk query dan mutasi │ ├── server.ts # File untuk setup Apollo Server dan konfigurasi GraphQL │ ├── schema # Definisi schema GraphQL │ ├── types # TypeScript types dan interfaces untuk GraphQL dan lainnya │ └── utils # Berisi helper dan fungsi utility ├── .env # File konfigurasi environment (Redis, JWT Secret, Database URL) ├── package.json # Metadata proyek dan dependensi └── tsconfig.json # Konfigurasi TypeScript
The output will be in the dist/ folder and ready to be deployed to the production server.
Preparing for Cloud Platforms
This project can be deployed to platforms such as Heroku, AWS, or DigitalOcean with the following steps:
- Push Code to Git Repository (GitHub, GitLab, or others).
- Set Environment Variables on the selected cloud platform (Redis, JWT Secret, URL Database).
- Deploy the project using commands or integrations from the cloud platform.
? Resource
- GraphQL Documentation
- Redis Documentation
- JWT Documentation
- Sequelize Documentation
By following the steps above, you can now run and develop GraphQL API applications using Redis, JWT, and Sequelize.
The above is the detailed content of Node.js Starter Project dengan GraphQL, Redis, JWT, dan Sequelize. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
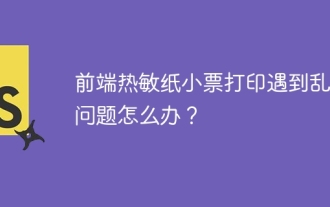
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
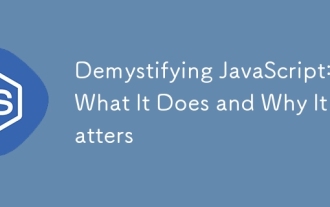
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
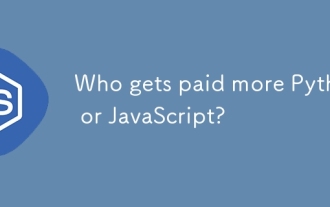
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
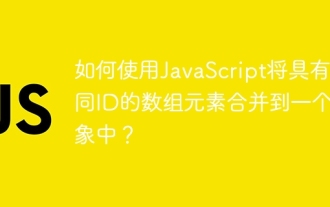
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
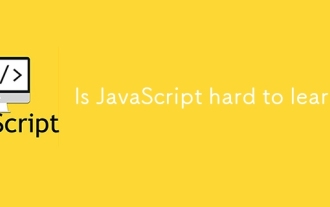
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
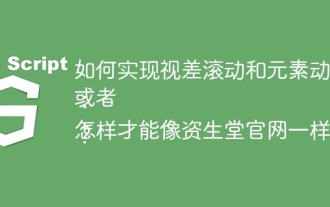
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
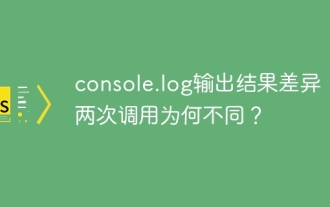
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
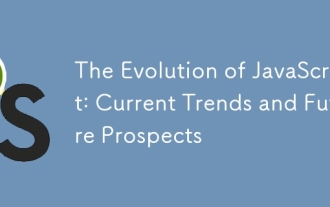
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
