Why are Virtual Destructors Essential for Abstract Classes?
Nov 13, 2024 am 08:02 AMThe Paramount Importance of Virtual Destructors in Abstract Classes
While declaring virtual destructors is a recommended practice for base classes in C , its significance extends even further for abstract classes, often serving as interfaces. Here are compelling reasons and an illustrative example:
Enforcing Proper Deallocation:
It's crucial for abstract classes to declare virtual destructors because they allow for proper deallocation of objects used through interface pointers. Without virtualization, deleting an object pointed to by an interface pointer results in undefined behavior, as the compiler may call the base class destructor instead of the derived class destructor. This can lead to memory leaks or crashes.
Example:
Consider the following code snippet:
class Interface { virtual void doSomething() = 0; }; class Derived : public Interface { Derived(); ~Derived() { // Important cleanup... } }; void myFunc() { Interface* p = new Derived(); delete p; // Undefined behavior, may call Interface::~Interface instead }
In this example, deleting p will invoke the base class destructor (Interface::~Interface), which is not aware of the cleanup actions performed in the derived class destructor (Derived::~Derived). This omission can result in memory leaks or unpredictable behavior.
Virtual Destructors Ensure Safe Deletion:
By declaring a virtual destructor in an abstract class, polymorphism is enabled. When an object pointed to by an interface pointer is deleted, the compiler will call the appropriate derived class destructor, ensuring that all necessary cleanup actions are executed.
Wrap-Up:
Virtual destructors are indispensable for abstract classes serving as interfaces. They guarantee the safe deletion of objects accessed through interface pointers, preventing memory leaks and undefined behavior.
The above is the detailed content of Why are Virtual Destructors Essential for Abstract Classes?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
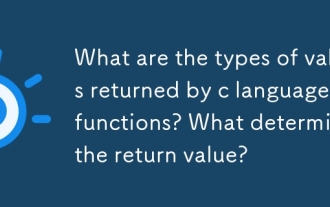
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
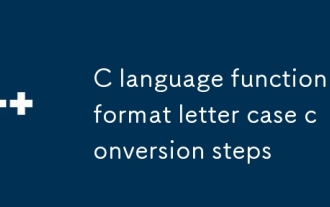
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
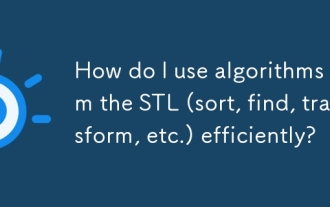
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
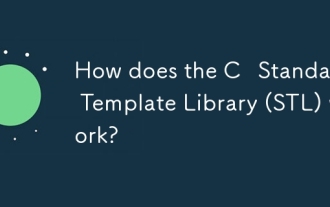
How does the C Standard Template Library (STL) work?
