How to Convert JSON Strings to HashMaps in Java Using the org.json Library?
Converting JSON Strings to HashMaps in Java Using the org.json Library
When working with JSON data in Java, a common task is to convert JSON strings into HashMaps to facilitate data manipulation and storage. This article provides a detailed explanation on how to achieve this conversion using the org.json library.
JSON Structure
Consider the following JSON string:
{ "name" : "abc", "email id" : ["[email protected]", "[email protected]", "[email protected]"] }
The goal is to convert this JSON string into a HashMap with keys matching the property names and values corresponding to their respective values.
HashMap Initialization
First, initialize a HashMap to store the converted data:
Map<String, Object> retMap = new HashMap<String, Object>();
Recursive Conversion
The conversion process involves recursively traversing the JSON object and converting nested structures as well. The following code snippet outlines the recursive conversion method:
public static MapjsonToMap(JSONObject json) throws JSONException { Map<String, Object> retMap = new HashMap<String, Object>(); if(json != JSONObject.NULL) { retMap = toMap(json); } return retMap; } public static Map toMap(JSONObject object) throws JSONException { Map map = new HashMap (); Iterator keysItr = object.keys(); while(keysItr.hasNext()) { String key = keysItr.next(); Object value = object.get(key); if(value instanceof JSONArray) { value = toList((JSONArray) value); } else if(value instanceof JSONObject) { value = toMap((JSONObject) value); } map.put(key, value); } return map; } public static List
This code recursively traverses the JSON object and handles nested structures, such as arrays and objects, ensuring that the final HashMap contains all the data in the JSON string.
Using the Jackson Library
Alternatively, you can utilize the Jackson library for converting JSON strings to HashMaps. This can be done with the following code:
import com.fasterxml.jackson.databind.ObjectMapper; Map<String, Object> mapping = new ObjectMapper().readValue(jsonStr, HashMap.class);
The above is the detailed content of How to Convert JSON Strings to HashMaps in Java Using the org.json Library?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










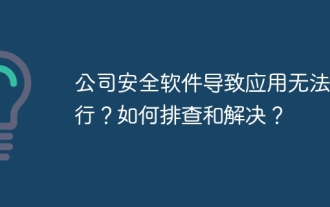
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
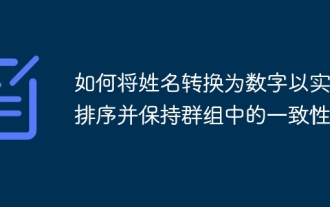
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
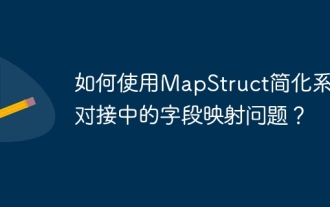
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
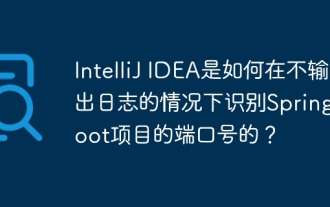
Start Spring using IntelliJIDEAUltimate version...
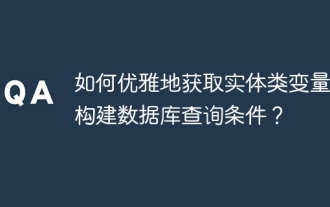
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
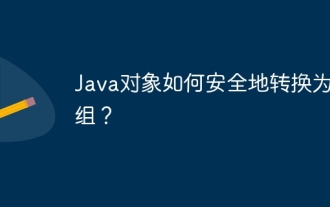
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
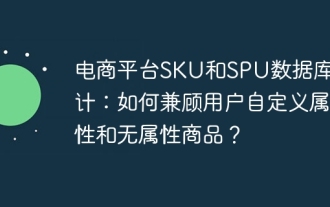
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
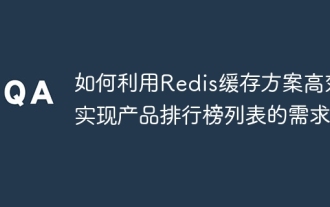
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
