How to Load Images Asynchronously in Java Swing to Prevent GUI Freezing?
Loading an Image Asynchronously
In the provided code, the image loading process may freeze the GUI, particularly when the image is large or the network connection is slow. To prevent this issue and keep the GUI responsive, we can utilize a background thread to load the image. This technique is referred to as asynchronous image loading.
Using SwingWorker for Asynchronous Loading
Java's javax.swing.SwingWorker class provides a convenient way to perform background tasks and update the GUI when they are complete. Here's how we can use it to asynchronously load an image:
import javax.swing.SwingWorker; import java.awt.image.BufferedImage; import javax.swing.JLabel; public class ImageLoader extends SwingWorker<BufferedImage, Void> { private final String imageUrl; private final JLabel label; public ImageLoader(String imageUrl, JLabel label) { this.imageUrl = imageUrl; this.label = label; } @Override protected BufferedImage doInBackground() throws Exception { // Load the image in the background BufferedImage image = ImageIO.read(new URL(imageUrl)); return image; } @Override protected void done() { try { BufferedImage image = get(); // Update the GUI with the loaded image in the EDT label.setIcon(new ImageIcon(image)); } catch (Exception e) { e.printStackTrace(); } } }
Integrating with Your Code
To integrate this solution with your code:
-
In the client_trackedbus class, create an instance of ImageLoader and execute it in the constructor:
public client_trackedbus( ... ) { // ... ImageLoader imageLoader = new ImageLoader("http://www.huddletogether.com/projects/lightbox2/images/image-2.jpg", label); imageLoader.execute(); // ... }
Copy after login -
In the displayMap method, remove the image loading code and simply set the label's visibility to true:
private void displayMap(...) { // ... label.setVisible(true); // ... }
Copy after login
By following these steps, the image loading process will be performed asynchronously, allowing the GUI to remain responsive while the image is being fetched.
The above is the detailed content of How to Load Images Asynchronously in Java Swing to Prevent GUI Freezing?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










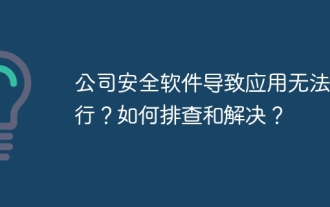
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
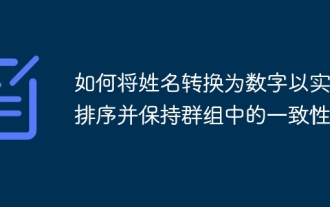
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
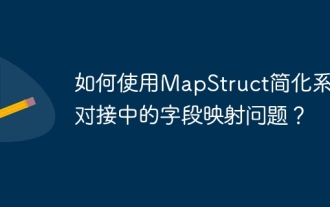
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
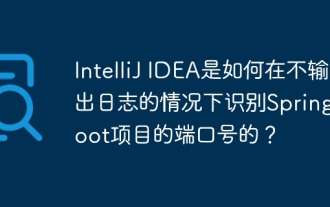
Start Spring using IntelliJIDEAUltimate version...
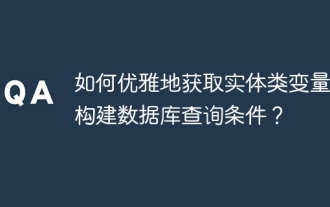
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
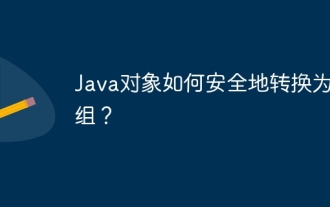
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
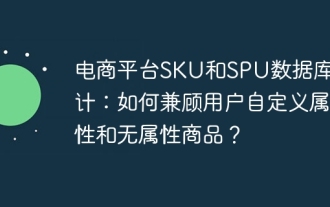
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
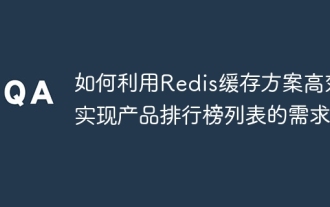
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
