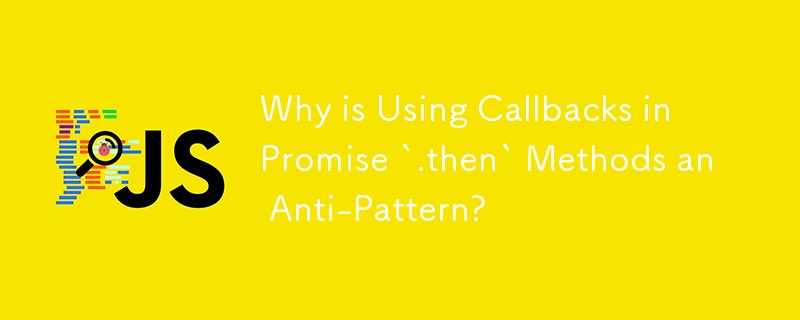
Using Callbacks in Promise .then Methods: An Anti-Pattern
The practice of providing a callback function to an AngularJS service as seen in the code snippet you provided may seem convenient, but it follows an anti-pattern. This approach disrupts the asynchronous programming flow and inversion of control intended by Promises.
Why is it an Anti-Pattern?
-
Interrupts Chaining: Promise .then methods allow you to chain multiple async operations. By using a callback, you lose the ability to easily continue processing the result of one operation in a subsequent one.
-
Inversion of Control: The original code inverts the control of execution by passing the callback from the consumer module to the provider module. This makes it harder to manage and debug the execution flow.
-
Unnecessary Promise Complexity: Promises provide a mechanism for handling asynchronous operations. By wrapping promises in callbacks, you introduce unnecessary complexity and obfuscate the intent of the code.
Refactoring Solution:
To refactor the code and eliminate these issues, you can use a plain .then method without a callback function:
var getTokens = function() {
return $http.get('/api/tokens');
};
Copy after login
In the consumer module, you can then directly use .then to chain operations:
yourModule.getTokens()
.then(function(response) {
// handle it
});
Copy after login
Benefits of the Refactored Code:
-
Maintains Chaining: By returning a Promise from the getTokens function, you can easily chain subsequent operations.
-
Preserves Control: The consumer module controls the execution of async operations without relying on callbacks.
-
Simplifies Code: The refactored code simplifies the asynchronous handling process, making it easier to understand and maintain.
The above is the detailed content of Why is Using Callbacks in Promise `.then` Methods an Anti-Pattern?. For more information, please follow other related articles on the PHP Chinese website!