


How can I return data from multiple database queries within a loop in JavaScript?
Issue with Returning Data from Multiple Database Queries in a Loop
When executing multiple database queries within a loop, it's essential to handle asynchronous operations properly to ensure that all data is retrieved before returning it to your client.
In the provided code, the getPrayerInCat function executes multiple MongoDB queries using the forEach callback. However, the code immediately returns undefined because the function does not wait for the results of all database queries to complete.
To resolve this issue, we must adhere to the following principles when working with promises:
- Every asynchronous function must return a promise.
- Create wrappers for functions that don't return promises to make them promise-compatible.
- Everything that interacts with an asynchronous result should go into a .then callback.
Using these principles, we can create a version of the getPrayerCount function that returns a promise:
function getPrayerCount(data2) { var id = data2.id; return find({prayerCat:id}) .then(function(prayer) { if (!prayer) data2.prayersCount = 0; else data2.prayersCount = prayer.length; return data2; }); }
To handle multiple asynchronous tasks and wait for their completion, we can use Q.all:
function getPrayerInCat(data) { var promises = data.map(getPrayerCount); // don't use forEach return Q.all(promises); }
By returning a promise from the getPrayerInCat function, we can wait for all queries to complete before returning the results.
The above is the detailed content of How can I return data from multiple database queries within a loop in JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
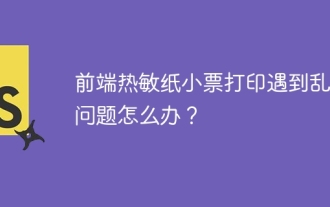
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
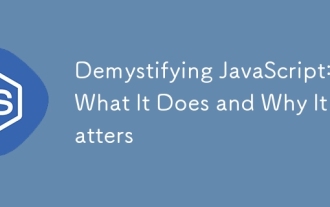
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
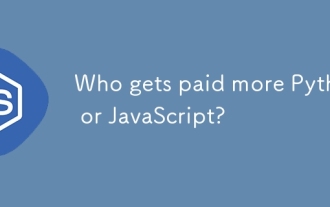
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
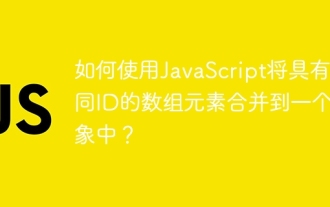
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
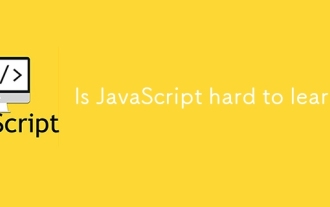
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
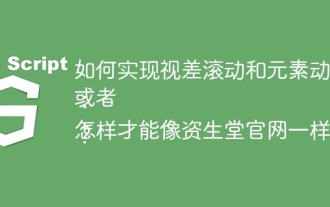
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
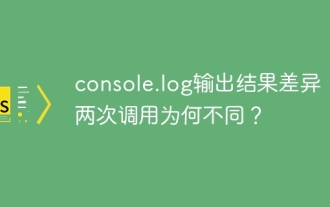
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
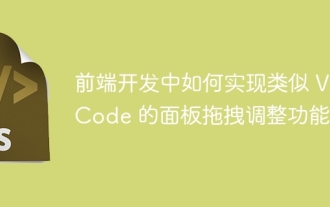
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
