


How to Build a Web Scraper in PHP Using cURL and Regular Expressions?
How to Implement a Web Scraper in PHP
Web scraping involves three primary steps:
- Sending a GET or POST request to a specific URL
- Receiving the HTML response
- Parsing the HTML to extract the desired text
PHP Built-In Functions for Web Scraping
cURL: a library for making HTTP requests and retrieving web content.
Regular Expressions: a powerful tool for parsing and matching text.
Useful PHP Resources for Web Scraping
Regular Expressions Tutorial: a comprehensive resource for learning regular expressions.
Regex Buddy: a helpful program for working with regular expressions, including code generation.
Example PHP Class for Web Scraping
Below is a simple PHP class that uses cURL to fetch webpages:
class Curl { // ... (code shown earlier) function get($url) { // ... (code shown earlier) return $this->request(); } } $curl = new Curl(); $html = $curl->get("http://www.google.com"); // Parse the HTML using regular expressions preg_match_all('/<title>(.*)<\/title>/', $html, $matches); echo $matches[1][0]; // Output: Google
This example retrieves the HTML from Google's homepage and extracts the page title using regular expressions.
Tips and Tricks
Use a Dedicated Library for Scraping: Specialized libraries like PHPQuery or Scrapy provide advanced features for web scraping.
Handle CAPTCHAs and other Anti-Scraping Techniques: Protect against common anti-scraping measures.
Respect Server Limits: Ensure you do not overload servers with excessive scraping.
Have Fun: Web scraping can be an exciting and rewarding skill to master.
The above is the detailed content of How to Build a Web Scraper in PHP Using cURL and Regular Expressions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


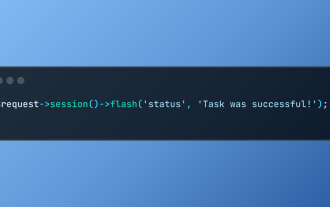
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
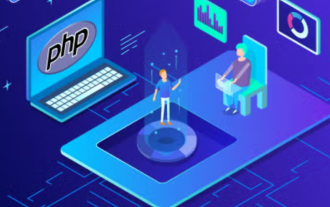
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
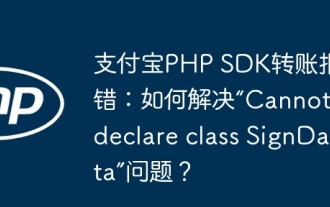
Alipay PHP...
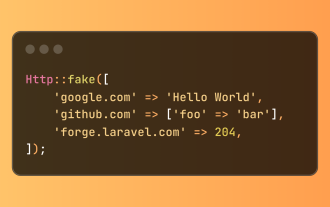
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
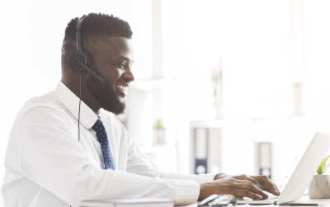
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
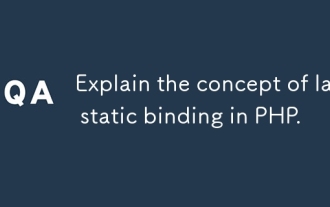
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
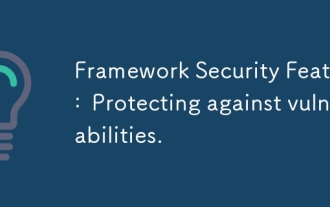
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
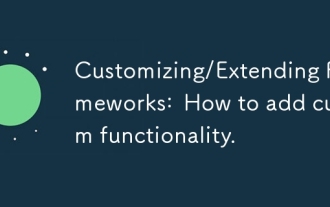
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
