How to Merge Firestore Queries Locally While Preserving Order?
Merging Firestore Queries Locally with Proper Ordering
In the absence of a logical OR operator in Firestore, merging two separate queries must be done locally to retrieve the desired results. To maintain proper ordering, consider using the Tasks.whenAllSuccess() method instead of nesting the second query within the success listener of the first.
Here's a code snippet demonstrating the recommended approach:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
The whenAllSuccess() method returns a Task that will be completed when all the provided tasks are successfully completed. The result of the Task is a list of objects, where each object represents the result of the corresponding task. In this case, the list will contain two elements, each representing the result of firstTask and secondTask respectively. The order of the elements in the list will match the order in which the tasks were specified to whenAllSuccess().
This approach ensures that the results are ordered based on the order of the tasks, allowing you to merge the results from multiple queries while preserving their proper sequence.
The above is the detailed content of How to Merge Firestore Queries Locally While Preserving Order?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










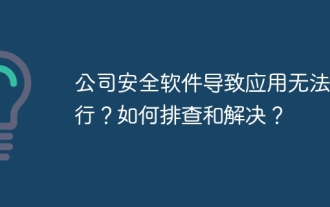
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
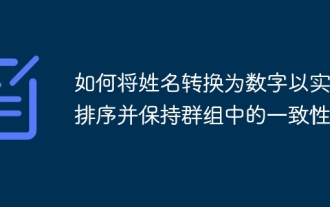
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
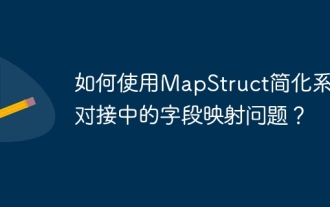
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
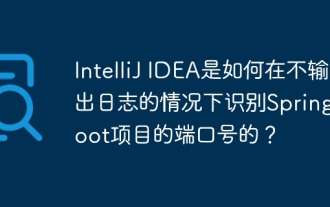
Start Spring using IntelliJIDEAUltimate version...
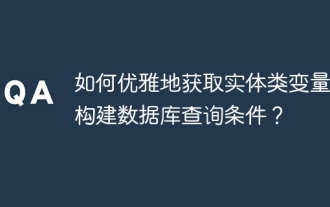
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
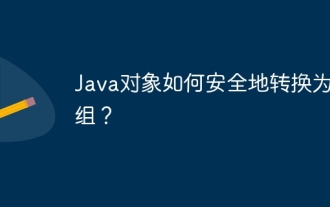
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
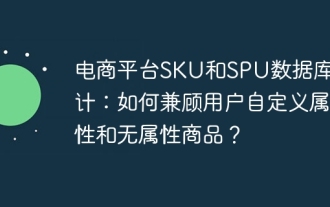
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
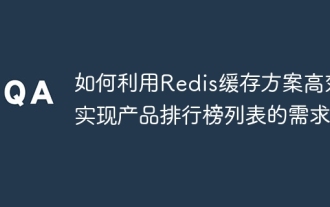
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
