


How do I 'delete' a struct in Go when garbage collection manages memory?
Understanding Object Removal in Go: A Case Study
In Go, structs serve as composite data types whose instances can store diverse information. Consider the following Person struct:
type Person struct { name string age int }
Now, if we instantiate this struct:
person1 := Person{name: "Name", age: 69}
Assigning nil to a struct object (e.g., person1 = nil) raises a type assignment error. This differs from the case of maps and slices where nil can be used.
Go's Garbage Collection Mechanism
Go adopts a garbage collection approach to memory management. The garbage collector automatically identifies unreachable objects and releases their allocated memory. This means that explicitly deleting objects is not possible or necessary in Go.
Strategies for Clearing and Overwriting Structs
Although deletion is not an option, structs can be cleared or overwritten by assigning another struct value, typically the zero value (an empty struct):
person1 := Person{name: "Name", age: 69} // work with person1 // Clear person1: person1 = Person{}
Note that this action does not genuinely free allocated memory for person1; the garbage collector will handle this when it becomes unreachable.
Handling Pointers to Structs
For pointers to Person (*Person), assigning nil (setting it to nil) will effectively clear the reference and its pointed object. This process leaves the garbage collector to release the pointed object's memory:
person1 := &Person{name: "Name", age: 69} // work with person1 // Clear person1: person1 = nil
Conclusion
Go's garbage collection mechanism handles object removal efficiently, eliminating the need for explicit deletion. Structs can be cleared by assigning them a zero value or setting pointers to nil, with the garbage collector ensuring proper memory management.
The above is the detailed content of How do I 'delete' a struct in Go when garbage collection manages memory?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










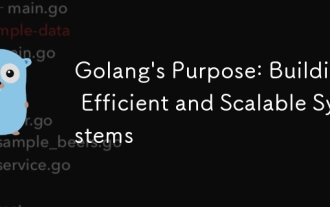
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
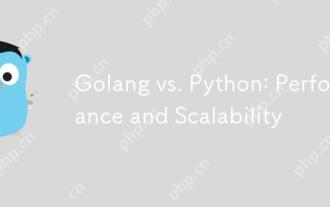
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
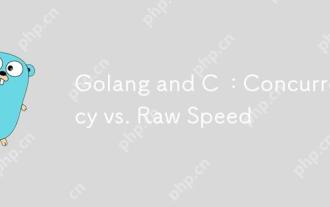
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
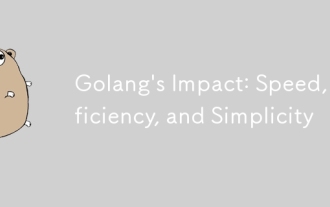
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
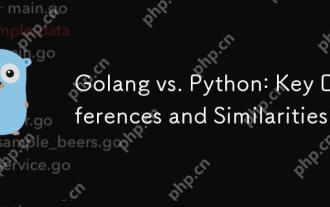
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
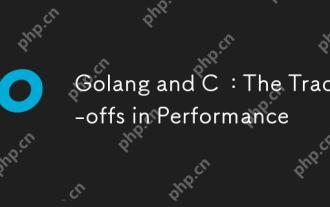
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
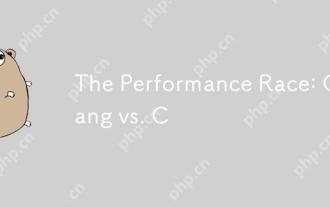
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
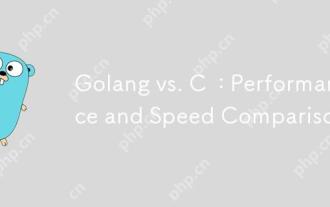
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
