


How to Pass Multiple Variables to a Spring MVC Controller Using Ajax Without a Backing Object?
Passing Multiple Variables to a Spring MVC Controller using Ajax
When using @RequestBody to pass multiple variables to a Spring MVC controller, it is not necessary to wrap them in a backing object. However, there are alternative approaches that can provide more flexibility or simplify the handling of JSON data.
Option 1: Use a Map
If you do not require strongly-typed parameters, you can use a Map
@RequestMapping(value = "/Test", method = RequestMethod.POST) @ResponseBody public boolean getTest(@RequestBody Map<String, String> json) { //json.get("str1") == "test one" }
This approach does not require a custom backing object and can handle JSON data with arbitrary keys.
Option 2: Use Jackson's ObjectNode
For more flexibility, you can bind to com.fasterxml.jackson.databind.node.ObjectNode to access the JSON data as a full JSON tree:
@RequestMapping(value = "/Test", method = RequestMethod.POST) @ResponseBody public boolean getTest(@RequestBody ObjectNode json) { //json.get("str1").asText() == "test one" }
This approach allows you to dynamically process the JSON data and extract values based on their JSON path.
Other Considerations:
- If strongly-typed parameters are required, you can create a custom POJO to represent the expected JSON structure and use @RequestBody to bind to it.
- For simple cases, using @RequestParam in the query string or @PathVariable in the request URI can be more convenient for passing individual variables.
The above is the detailed content of How to Pass Multiple Variables to a Spring MVC Controller Using Ajax Without a Backing Object?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










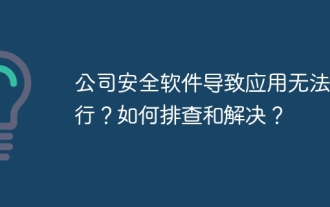
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
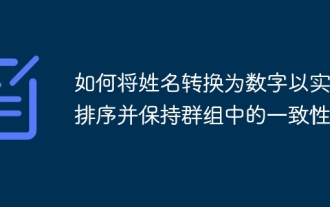
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
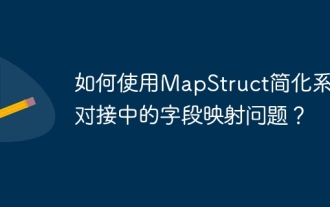
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
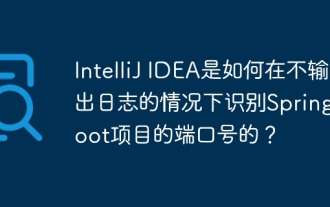
Start Spring using IntelliJIDEAUltimate version...
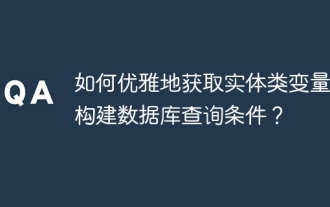
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
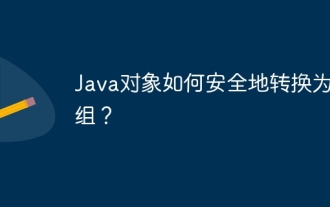
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
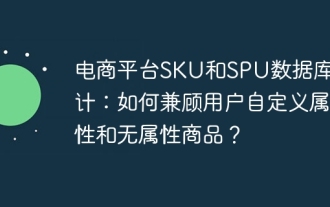
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
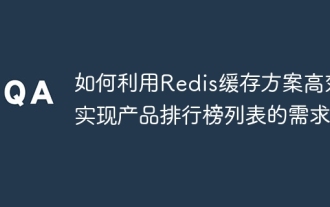
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
