


How can I efficiently extract a substring between two given substrings in Python?
Find String between Two Substrings Efficiently
Extracting a specific substring between two given substrings can be a common requirement in various coding scenarios. Consider a situation where you need to isolate the text within delimiters like '123' and 'abc' to obtain 'STRING' from '123STRINGabc'.
While a manual string slicing approach like the one provided ((s.split(start))[1].split(end)[0]) works, it falls short in terms of efficiency and Pythonic elegance.
Improved Solution using Regular Expressions
A highly effective solution leverages regular expressions (regex) in Python. Regex offers a concise and versatile means to perform pattern matching and extraction tasks. For our purpose, we can utilize the following regex pattern:
asdf=5;(.*)123jasd
- Matching Start Delimiter: asdf=5; ensures that the pattern starts with the designated left delimiter.
- Capturing Group: The parentheses (.*) capture everything in between the start and end delimiters.
- Matching End Delimiter: 123jasd verifies that the pattern ends with the designated right delimiter.
To execute the regex search on our input string, we can use the following code:
import re s = 'asdf=5;iwantthis123jasd' result = re.search('asdf=5;(.*)123jasd', s) print(result.group(1)) # Output: 'iwantthis'
The re.search() function scans the string for the specified pattern and returns a Match object. The group(1) method then retrieves the captured substring, which is the text between the delimiters.
Advantages of Using Regex
This regex-based approach offers several benefits:
- Precision: It accurately extracts the desired substring without any manual labor.
- Efficiency: Regex leverages sophisticated algorithms to match patterns swiftly, resulting in time optimization.
- Conciseness: The code is compact and easy to understand, promoting Pythonic best practices.
- Robustness: Regex handles cases where the string extends beyond the delimiters seamlessly.
In conclusion, using regular expressions is an elegant and efficient solution for finding substrings between two given substrings in Python.
The above is the detailed content of How can I efficiently extract a substring between two given substrings in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










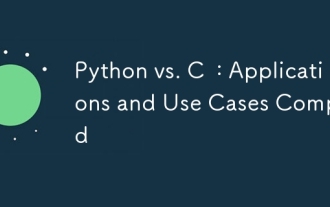
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
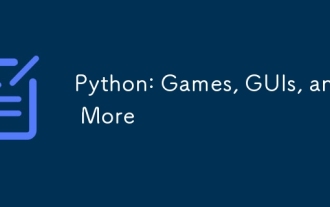
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
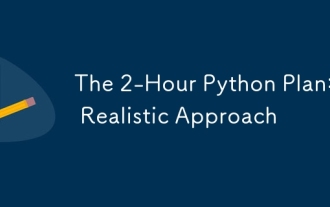
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
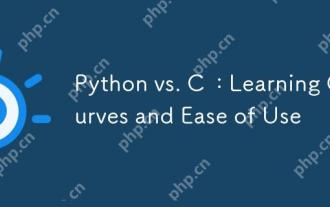
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
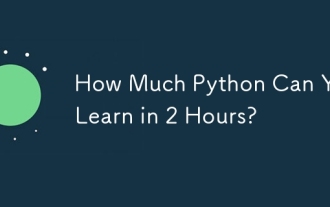
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
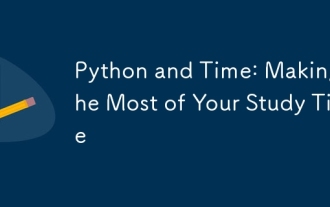
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
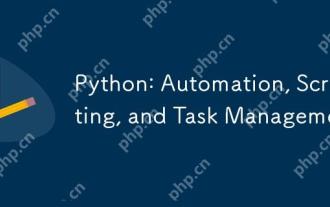
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
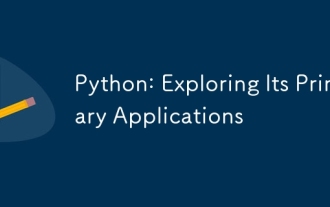
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
