


How can I achieve string interpolation in Python like Ruby?
Python's Equivalent to Ruby's String Interpolation
Ruby's string interpolation, which allows for easy inclusion of expressions within a string, has a Python equivalent in various forms.
Format String Interpolation (f-strings)
Python 3.6 and later introduced "f-strings," which enable literal string interpolation. Expressions can be directly inserted using the syntax:
name = "Spongebob Squarepants" print(f"Who lives in a Pineapple under the sea? {name}.")
String Interpolation with the % Operator
Prior to Python 3.6, string interpolation can be achieved using the % operator. The first operand is the string to be interpolated, while the second operand can be a mapping that matches field names to values. For example:
name = "Spongebob Squarepants" print("Who lives in a Pineapple under the sea? %(name)s." % locals())
String Interpolation with .format() Method
Recent Python versions also provide the .format() method for string interpolation:
name = "Spongebob Squarepants" print("Who lives in a Pineapple under the sea? {name!s}.".format(**locals()))
String.Template Class
Another option is to use the string.Template class:
tmpl = string.Template("Who lives in a Pineapple under the sea? $name.") print(tmpl.substitute(name="Spongebob Squarepants"))
The above is the detailed content of How can I achieve string interpolation in Python like Ruby?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


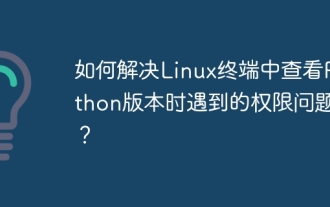
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
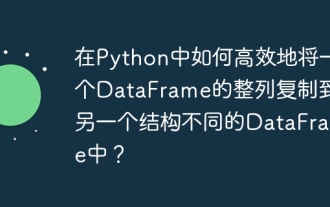
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
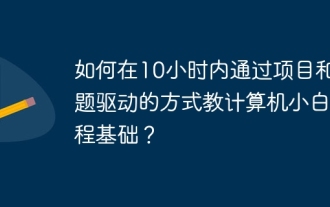
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
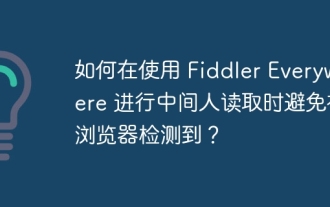
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
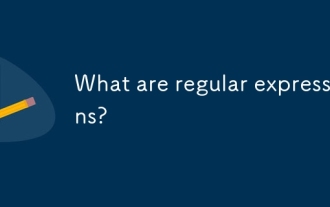
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
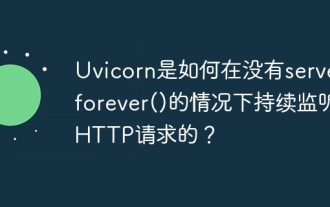
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
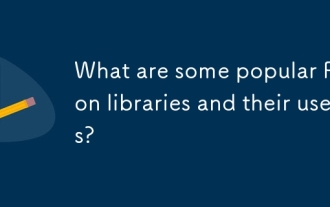
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
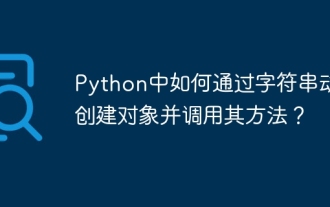
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
