


How to Capture HTTP Requests in Python Applications Using the Requests Library?
Inspecting HTTP Requests in Python Applications
Identifying the source of errors during API calls can be challenging, especially when the error response lacks specific details. To resolve such issues, API providers often require the entire HTTP request, including headers. This article presents a convenient approach for capturing these requests using the popular requests library.
Using Logging to Capture Requests
Recent versions of requests (1.x and above) offer a simple logging mechanism to capture HTTP requests. By enabling debugging at the http.client level, we can log both the request (including headers and body) and the response (including headers).
Implementation
The following code snippet demonstrates how to enable HTTP request logging:
import requests import logging # Enable debugging at http.client level http_client.HTTPConnection.debuglevel = 1 # Initialize and configure logging logging.basicConfig() logging.getLogger().setLevel(logging.DEBUG) requests_log = logging.getLogger("requests.packages.urllib3") requests_log.setLevel(logging.DEBUG) requests_log.propagate = True # Make an HTTP request requests.get('https://httpbin.org/headers')
By executing this code, we enable request logging and store the logged data in the requests_log variable. We can then access the request headers and body from this variable as needed.
Example Output
The following is an example of the debug output generated by the logging mechanism:
send: 'GET /headers HTTP/1.1\r\nHost: httpbin.org\r\nAccept-Encoding: gzip, deflate, compress\r\nAccept: */*\r\nUser-Agent: python-requests/1.2.0 CPython/2.7.3 Linux/3.2.0-48-generic\r\n\r\n'
This output contains the entire HTTP request, including the HTTP method, URI, headers, and request body (if present). By providing this information to API providers, you can facilitate the identification and resolution of errors.
The above is the detailed content of How to Capture HTTP Requests in Python Applications Using the Requests Library?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
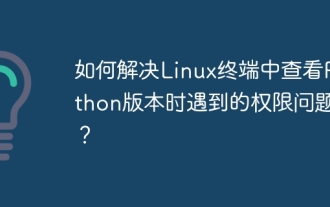
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
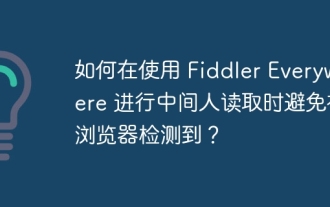
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
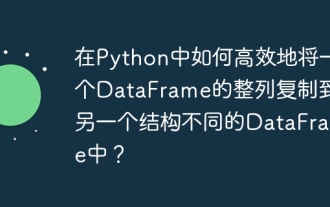
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
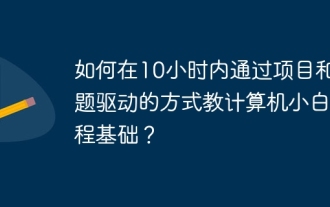
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
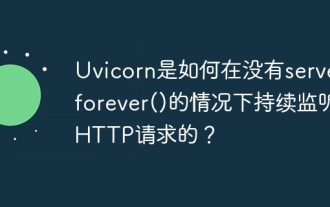
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
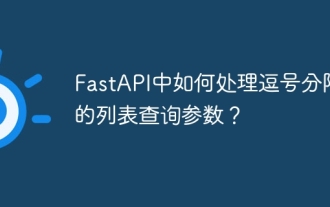
Fastapi ...
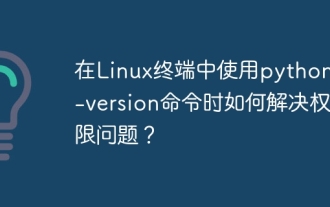
Using python in Linux terminal...
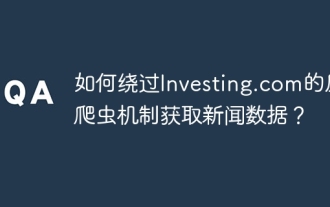
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
