


How can I dynamically modify CSS class property values using JavaScript?
Dynamically Modifying CSS Class Property Values Using JavaScript / jQuery
You have encountered a situation where you wish to dynamically assign a value to a CSS style, specifically for a class property that affects multiple elements in a slideshow. This requires directly modifying the CSS stylesheet, rather than simply adjusting element attributes.
Using a CSS Editing Library
While some may suggest otherwise, it is possible and efficient to edit CSS stylesheets with JavaScript. One recommended approach is to utilize the jss library.
import jss from 'jss'; const stylesheet = jss.createStyleSheet({ myClass: { fontSize: '2rem', }, });
Modifying a Single Item
To change the CSS class property values for a specific element, target it with a query selector and use the rule() method.
const element = document.querySelector('.myClass'); stylesheet.rule('.myClass').style({ color: 'red', });
Modifying Multiple Items Simultaneously
To modify the CSS class property values for all elements in a group, loop through them using the class name and the apply() method.
const elements = document.querySelectorAll('.myClass'); stylesheet.rule('.myClass').unfolded().apply(elements);
By utilizing a CSS editing library and employing efficient techniques, you can dynamically modify CSS class property values on the fly with JavaScript, eliminating the need to specify individual property values for each element.
The above is the detailed content of How can I dynamically modify CSS class property values using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










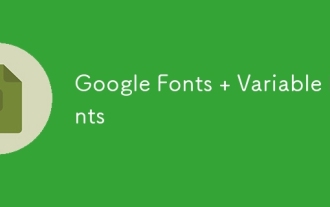
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
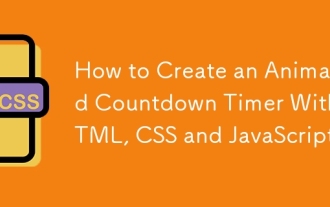
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
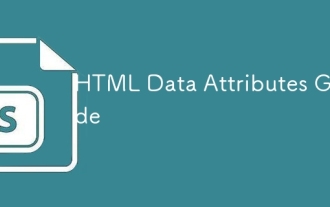
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
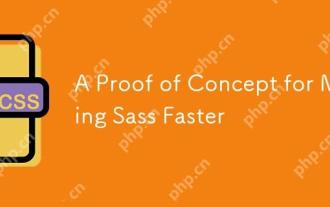
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
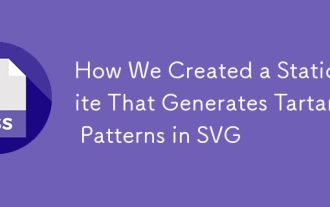
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
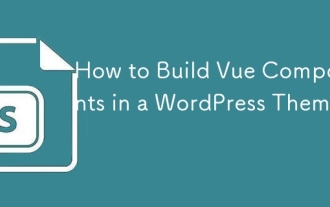
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
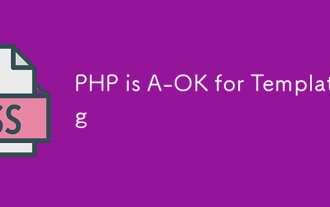
PHP templating often gets a bad rap for facilitating subpar code — but that doesn't have to be the case. Let’s look at how PHP projects can enforce a basic
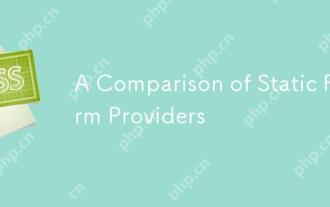
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
