


How to Access Package-Level Constants or Variables When a Local Variable with the Same Name Exists in Go?
Referencing Constants or Package-Level Variables over Function-Level Variables
In Go, the scope of variables determines their accessibility within different blocks of code. When local variables and top-level constants or package-level variables share the same name, a common issue arises: how to refer to the constant or package-level variable instead of the local one?
The Problem
Consider the following Go program:
package main import "fmt" const name = "Yosua" // or var name string = "James" func main() { name := "Jobs" fmt.Println(name) }
This program declares a constant name at the package level, but within the main function, another variable named name is declared at the function level. When the program runs, it prints "Jobs," which is the value of the local function-level variable name. How can you access the package-level constant name instead?
No Direct Reference
Unfortunately, Go does not provide a direct way to refer to top-level identifiers within the scope of a block where a local variable with the same name exists. According to the Go specification for Declarations and Scope, a locally declared identifier takes precedence within its scope.
Workaround Solutions
To access both the top-level variable and the local variable, you can use different names or employ one of the following workarounds:
Saving the Top-Level Value First
cname := name name := "Jobs" fmt.Println(name) fmt.Println(cname)
This method saves the value of the top-level constant or variable before creating the local variable.
Using a Function to Access the Top-Level Value
func getName() string { return name } name := "Jobs" fmt.Println(name) fmt.Println(getName())
This approach provides an alternative way to access the top-level variable by defining a function that returns its value.
Output
Both methods return the same output:
Jobs Yosua
This demonstrates that you can access the top-level variable while still using a local variable with the same name by using one of these workarounds. However, it's important to remember that local variables take precedence over top-level identifiers within their scope.
The above is the detailed content of How to Access Package-Level Constants or Variables When a Local Variable with the Same Name Exists in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


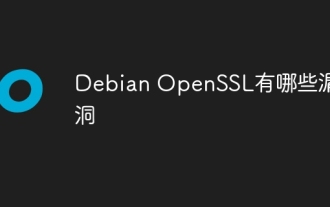
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
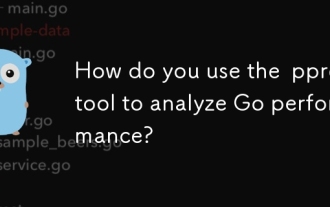
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
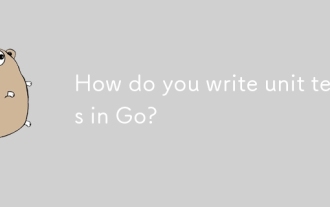
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
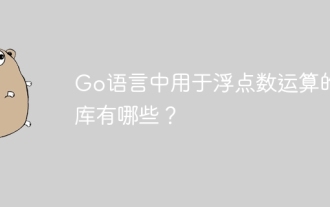
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
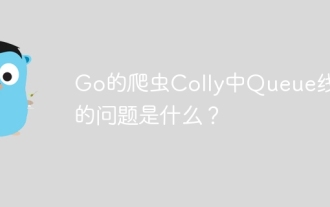
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
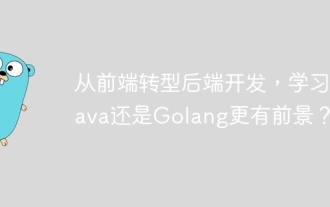
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
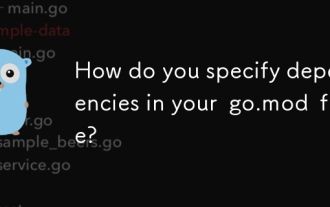
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
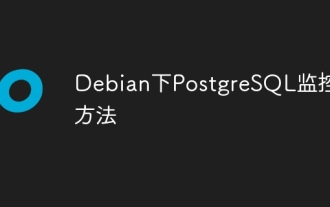
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
