


How to Perform Search and Replace on HTML Content While Ignoring HTML Tags?
Ignoring HTML Tags in preg_replace
When using preg_replace to perform search and replace operations on strings that contain HTML, it is often desirable to ignore the HTML tags and only modify the actual text content. However, this can be challenging using regular expressions alone, as they are not well-suited for parsing HTML.
One alternative approach is to utilize DOMDocument and DOMXPath to handle the HTML structure. By leveraging XPath queries, it is possible to locate text nodes within the HTML document that match the search criteria, and then wrap those nodes with the desired HTML elements without affecting the rest of the HTML tags.
For example, consider the following code snippet which avoids HTML tag interference:
$str = '...'; // HTML document $search = 'text to highlight'; $doc = new DOMDocument; $doc->loadXML($str); $xp = new DOMXPath($doc); $anchor = $doc->getElementsByTagName('body')->item(0); if (!$anchor) { throw new Exception('Anchor element not found.'); } // XPath query to locate text nodes containing the search text $r = $xp->query('//*[contains(., "'.$search.'")]/*[FALSE = contains(., "'.$search.'")]/..', $anchor); if (!$r) { throw new Exception('XPath failed.'); } // Process search results foreach($r as $i => $node) { $textNodes = $xp->query('.//child::text()', $node); $range = new TextRange($textNodes); // Identify matching text node ranges $ranges = array(); while (FALSE !== $start = $range->indexOf($search)) { $base = $range->split($start); $range = $base->split(strlen($search)); $ranges[] = $base; } // Wrap matching text nodes with HTML elements foreach($ranges as $range) { foreach($range->getNodes() as $node) { $span = $doc->createElement('span'); $span->setAttribute('class', 'search_highlight'); $node = $node->parentNode->replaceChild($span, $node); $span->appendChild($node); } } } echo $doc->saveHTML();
This code utilizes XPath queries to locate text nodes that contain the search term, and then creates a TextRange class to manage the subranges within the text nodes. Each matching range is then wrapped within a span element with a custom class, which can be used for highlighting or other purposes.
By employing DOMDocument and DOMXPath instead of relying solely on regular expressions, this approach provides a more efficient and reliable way to ignore HTML tags when performing search and replace operations on HTML content.
The above is the detailed content of How to Perform Search and Replace on HTML Content While Ignoring HTML Tags?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




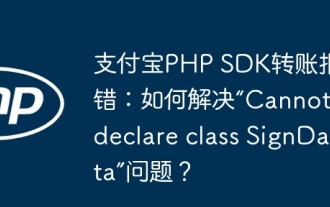
Alipay PHP...
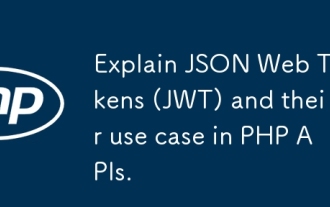
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
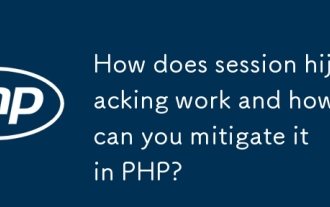
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
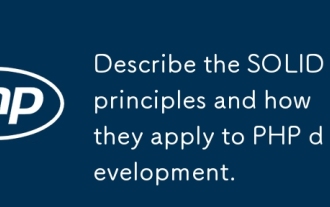
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
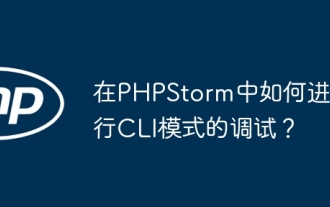
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
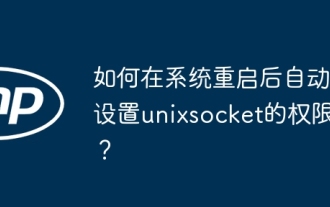
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
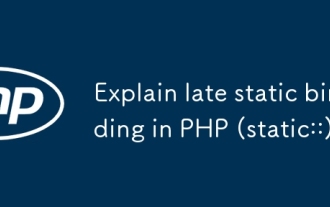
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
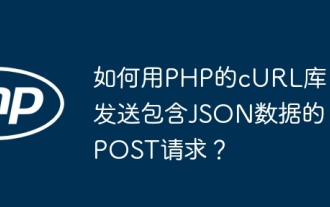
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
