


How to Force Browser Reflow for CSS Transitions: A Guide to Effective Animations
Browser Reflow Problem in CSS Transition
When transitioning DOM elements using CSS3, it's often necessary to force a browser reflow to trigger animations. In cases where modifying multiple CSS properties in sequence results in unexpected behavior, the browser may optimize the changes, leading to the absence of animations.
To resolve this issue, it's essential to understand the concept of reflow. A reflow occurs when the browser recalculates the layout of a portion of the document tree, which is triggered by changes to the size or position of block-level elements.
One technique to force reflow involves accessing the offsetHeight property of an element after modifying its styles. This can be accomplished through the following function:
function reflow(elt){ console.log(elt.offsetHeight); }
By calling this function after updating the element's CSS, a reflow can be triggered, allowing the transition to take effect. Here is a modified example of the code from the question that uses this technique:
ul.style.transition = 'none 0s linear 0s'; ul.style.left = '-600px'; reflow(ul); ul.style.transition = 'all 0.2s ease-out'; ul.style.left = '0px';
Another option is to use the void() operator, which prevents the optimizer from skipping the property access:
void(elt.offsetHeight);
This technique is effective as void is a unary operator that evaluates the expression and then discards its result, ensuring that any side effects of accessing the property are executed.
The above is the detailed content of How to Force Browser Reflow for CSS Transitions: A Guide to Effective Animations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


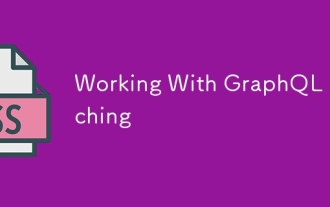
If you’ve recently started working with GraphQL, or reviewed its pros and cons, you’ve no doubt heard things like “GraphQL doesn’t support caching” or
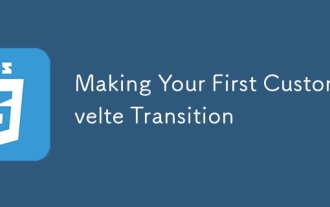
The Svelte transition API provides a way to animate components when they enter or leave the document, including custom Svelte transitions.
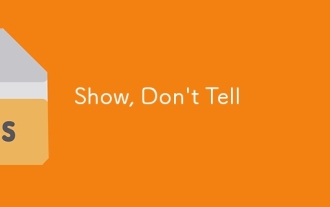
How much time do you spend designing the content presentation for your websites? When you write a new blog post or create a new page, are you thinking about
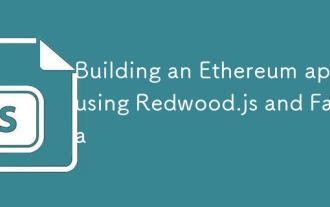
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
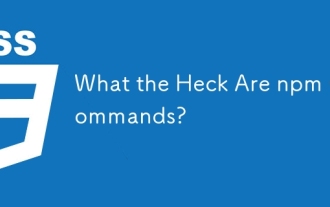
npm commands run various tasks for you, either as a one-off or a continuously running process for things like starting a server or compiling code.
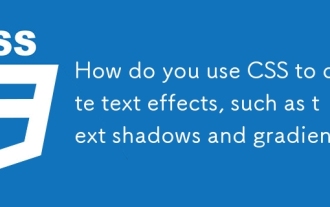
The article discusses using CSS for text effects like shadows and gradients, optimizing them for performance, and enhancing user experience. It also lists resources for beginners.(159 characters)
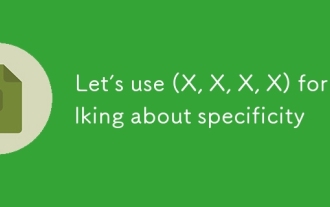
I was just chatting with Eric Meyer the other day and I remembered an Eric Meyer story from my formative years. I wrote a blog post about CSS specificity, and
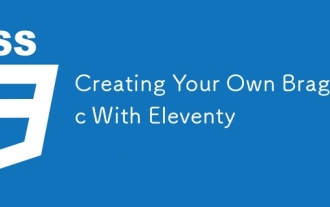
No matter what stage you’re at as a developer, the tasks we complete—whether big or small—make a huge impact in our personal and professional growth.
