


How Can I Programmatically Get the String Representation of a Go Type?
Programmatically Obtaining Type String Representation
When working with types in Go, it's essential to retrieve their string representations accurately. Manually instantiating the type or converting an interface to a string may not always be ideal. Here's a more robust and flexible approach:
Utilizing the reflect package, you can delve into the structure of types. By using a typed nil pointer value, you avoid unnecessary allocations while accessing the type descriptor. Navigating through the reflect.Type interface, you can access the base type (or element type) descriptor of the pointer.
import "reflect" type ID uuid.UUID func main() { t := reflect.TypeOf((*ID)(nil)).Elem() name := t.Name() fmt.Println(name) // Output: "ID" }
In the above example, the reflect package is employed to derive the string representation of the type ID, which is "ID."
Note: Keep in mind that for unnamed types, Type.Name() may return an empty string. However, for type declarations utilizing the type keyword, the name will be non-empty. Nonetheless, this technique provides a highly effective means to obtain the string representation of types dynamically, making it invaluable for refactoring and introspection tasks.
The above is the detailed content of How Can I Programmatically Get the String Representation of a Go Type?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
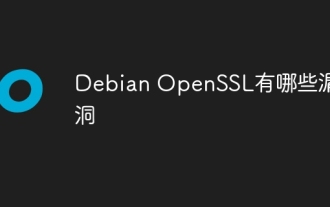
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
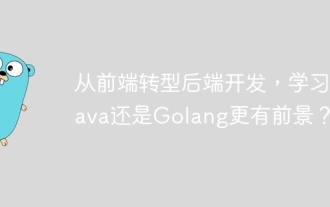
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
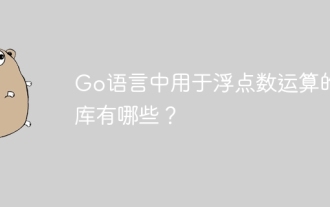
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
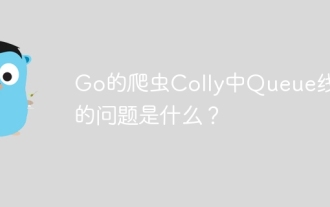
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
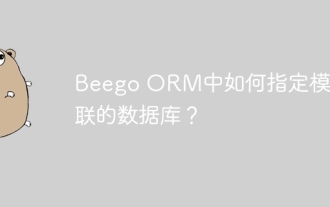
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
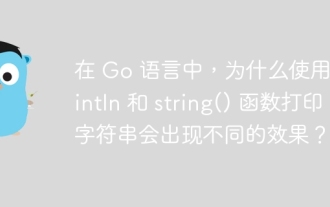
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
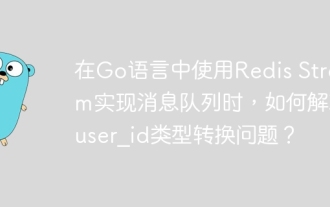
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
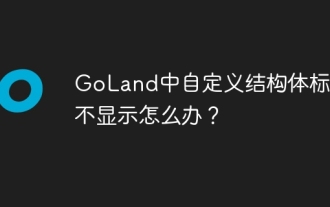
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
