How can I map MySQL\'s bit(1) type to a Go type using Beego\'s ORM?
MySQL's Bit Type: A Perfect Match with Go's Custom Bool
Question:
In a database table with a bit(1) type column, how can I map it to a Go type using Beego's ORM?
Answer:
Since Go doesn't have a built-in type for bit(1), the recommended approach is to create a custom bool type.
Custom Bool for MySQL's Bit(1)
To solve this issue, a custom bool type can be created to handle the bit(1) column.
type BitBool bool // Value converts BitBool to a bitfield (BIT(1)) for MySQL storage. func (b BitBool) Value() (driver.Value, error) { if b { return []byte{1}, nil } else { return []byte{0}, nil } } // Scan converts the incoming bitfield from MySQL into a BitBool. func (b *BitBool) Scan(src interface{}) error { v, ok := src.([]byte) if !ok { return errors.New("bad []byte type assertion") } *b = v[0] == 1 return nil }
Usage in Go Struct
To use the custom BitBool type in a Go struct, simply declare it as the type for the corresponding field:
type BaseModel struct { Id string `orm:"pk";form:"id"` CreatedTime time.Time `orm:"auto_now_add;type(datetime)";form:"-"` UpdatedTime time.Time `orm:"auto_now;type(datetime)";form:"-"` Deleted BitBool `form:"-"` }
By using a custom BitBool type, you can map MySQL's bit(1) type to a Go type that handles the bit manipulation appropriately, avoiding the errors that arise from using the default bool type.
The above is the detailed content of How can I map MySQL\'s bit(1) type to a Go type using Beego\'s ORM?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
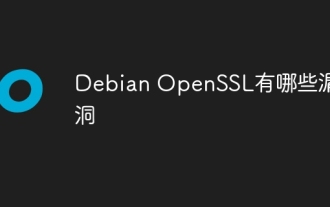
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
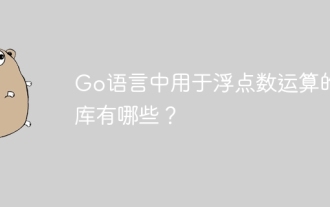
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
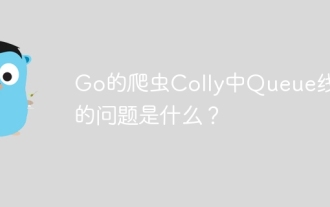
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
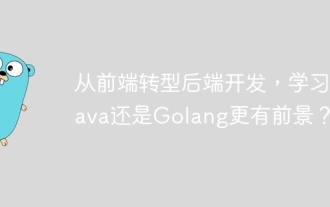
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
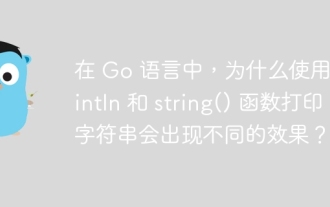
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
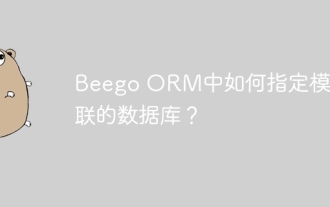
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
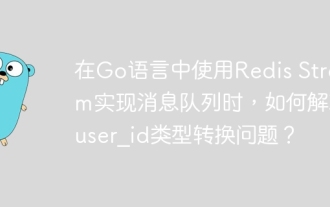
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
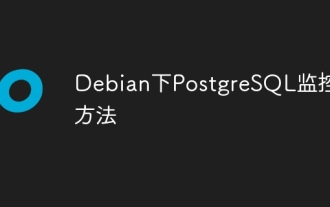
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
