How to Group Data by Team Using Angular\'s groupBy Filter?
Nov 17, 2024 am 11:59 AMGroup Data with Angular Filter: A Comprehensive Guide
Grouping data into meaningful categories is a common task in programming, and Angular provides a powerful filtering mechanism to facilitate this. This article demonstrates how to use Angular's groupBy filter to organize a list of players into teams.
Problem:
You have a dataset of players with their respective teams. You need to filter this dataset to display players grouped by their teams.
Example Dataset:
players = [ { name: 'Gene', team: 'team alpha' }, { name: 'George', team: 'team beta' }, { name: 'Steve', team: 'team gamma' }, { name: 'Paula', team: 'team beta' }, { name: 'Scruath', team: 'team gamma' } ];
Expected Output:
<li>team alpha <ul> <li>Gene</li> </ul> </li> <li>team beta <ul> <li>George</li> <li>Paula</li> </ul> </li> <li>team gamma <ul> <li>Steve</li> <li>Scruath</li> </ul> </li>
Solution:
To achieve this grouping, Angular provides the groupBy filter from its angular.filter module. This filter takes a property as an argument and returns an object where the keys are the unique values of that property, and the values are arrays of the objects that share that property value.
In our case, we want to group players by their team property. Here's how we can do it:
JavaScript:
$scope.players = players; // Assign the dataset to a scope variable
HTML:
<ul ng-repeat="(team, players) in players | groupBy: 'team'"> <li>{{team}} <ul> <li ng-repeat="player in players">{{player.name}}</li> </ul> </li> </ul>
By combining the groupBy filter with ng-repeat, we can create a hierarchical view of the data, where each team is displayed as a list item, and the players belonging to that team are listed as nested list items. The result is an organized and easy-to-understand representation of the grouped data.
Note:
To use the angular.filter module, it must be added as a dependency in your Angular module.
The above is the detailed content of How to Group Data by Team Using Angular\'s groupBy Filter?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
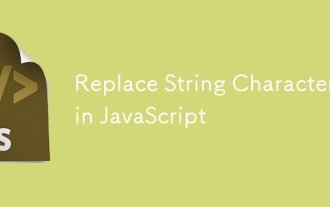
Replace String Characters in JavaScript
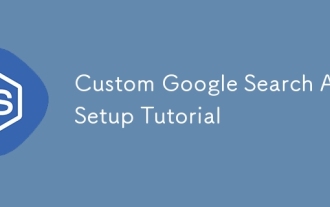
Custom Google Search API Setup Tutorial
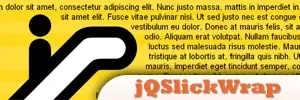
8 Stunning jQuery Page Layout Plugins
