


How can Selenium be integrated with Scrapy to handle dynamic web pages?
Integrating Selenium with Scrapy for Dynamic Web Pages
Introduction
Scrapy is a powerful web scraping framework, but it faces limitations when encountering dynamic web pages. Selenium, an automated web browser testing tool, can fill this gap by simulating user interactions and rendering page content. Here's how to integrate Selenium with Scrapy to handle dynamic web pages.
Selenium Integration Options
There are two primary options for integrating Selenium with Scrapy:
-
Option 1: Call Selenium in Scrapy Parser
- Initiate a Selenium session within the Scrapy parser method.
- Use Selenium to navigate and interact with the page, extracting data as needed.
- This option provides fine-grained control over Selenium's operation.
-
Option 2: Use scrapy-selenium Middleware
- Install the scrapy-selenium middleware package.
- Configure the middleware to handle specific requests or all requests.
- The middleware will automatically render pages using Selenium before they are processed by Scrapy's parsers.
Scrapy Spider Example with Selenium
Consider the following Scrapy spider that uses the first integration option:
class ProductSpider(CrawlSpider): name = "product_spider" allowed_domains = ['example.com'] start_urls = ['http://example.com/shanghai'] rules = [ Rule(SgmlLinkExtractor(restrict_xpaths='//div[@id="productList"]//dl[@class="t2"]//dt'), callback='parse_product'), ] def parse_product(self, response): self.log("parsing product %s" % response.url, level=INFO) driver = webdriver.Firefox() driver.get(response.url) # Perform Selenium actions to extract product data product_data = driver.find_element_by_xpath('//h1').text driver.close() # Yield extracted data as a scrapy Item yield {'product_name': product_data}
Additional Examples and Alternatives
-
For pagination handling on eBay using Scrapy Selenium:
class ProductSpider(scrapy.Spider): # ... def parse(self, response): self.driver.get(response.url) while True: # Get next page link and click it next = self.driver.find_element_by_xpath('//td[@class="pagn-next"]/a') try: next.click() # Scrape data and write to items except: break
Copy after login - Alternative to Selenium: Consider using ScrapyJS middleware for dynamic page rendering (see example in the provided link).
By leveraging Selenium's capabilities, you can enhance the functionality of your Scrapy crawler to handle dynamic web pages effectively.
The above is the detailed content of How can Selenium be integrated with Scrapy to handle dynamic web pages?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




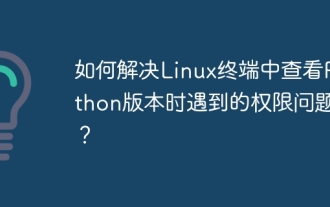
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
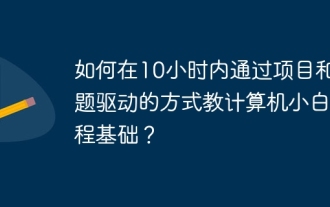
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
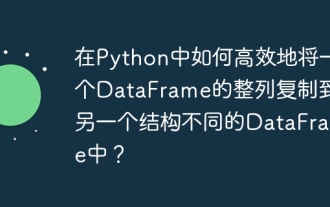
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
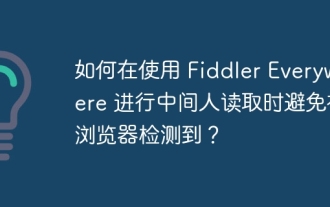
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
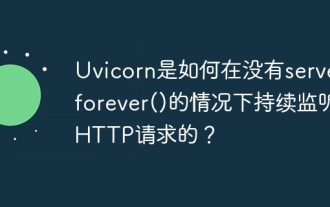
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
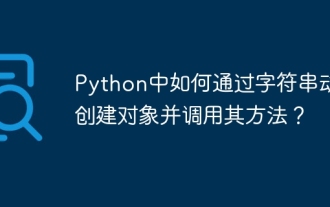
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
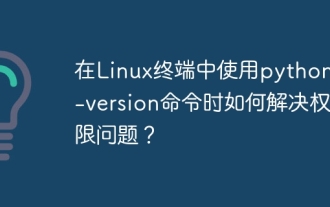
Using python in Linux terminal...
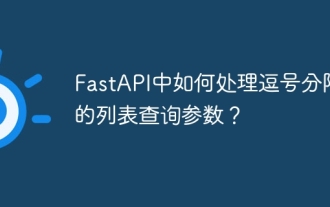
Fastapi ...
