How to Perform Case-Insensitive String Search in Go?
Case-Insensitive String Search in Go
Searching through text for specific words is a common task in programming. However, in real-world applications, these words may appear in various cases. To account for this, performing case-insensitive searches becomes essential. In this article, we will explore how to search for strings in a case-insensitive manner in Go.
Solution: Using the strings.EqualFold() Function
Go provides the strings.EqualFold() function, specifically designed for case-insensitive string comparisons. It compares two strings and ignores any differences in case between lowercase and uppercase letters.
Consider the following code snippet:
import ( "fmt" "strings" ) func main() { fmt.Println(strings.EqualFold("UpdaTe", "update")) fmt.Println(strings.EqualFold("ÑOÑO", "ñoño")) }
Both comparisons will return true because the strings.EqualFold() function disregards the differences in character casing.
Performance Considerations
It's important to note that case-insensitive string comparisons are computationally more expensive than case-sensitive comparisons. While the performance penalty is negligible for small datasets, it's advisable to consider optimizing the search for large volumes of data or time-sensitive applications.
Conclusion
The strings.EqualFold() function in Go provides a convenient and efficient way to perform case-insensitive string searches. By utilizing this function, you can ensure accurate search results regardless of the casing of the input strings.
The above is the detailed content of How to Perform Case-Insensitive String Search in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


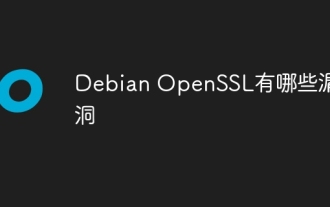
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
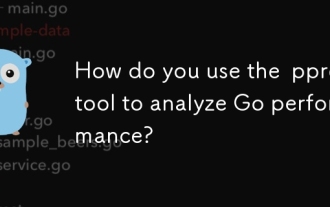
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
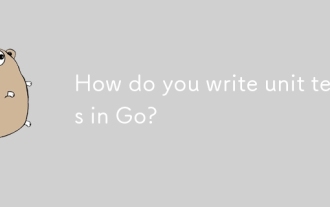
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
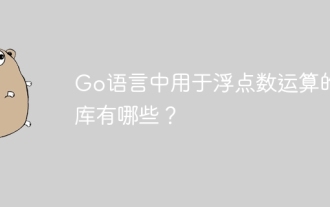
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
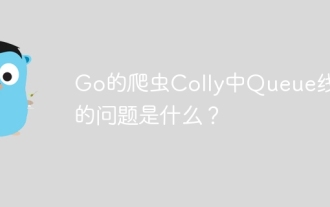
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
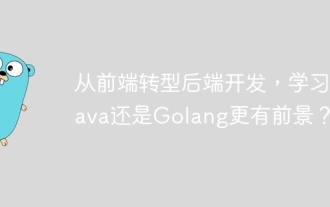
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
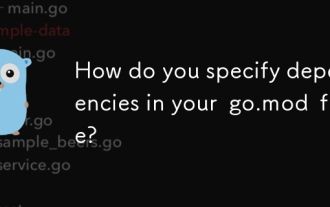
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
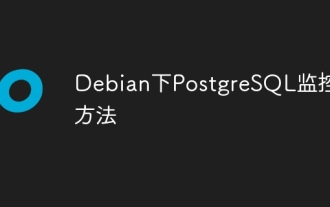
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
