


What are the differences between `IteratorIterator` and `RecursiveIteratorIterator` in PHP?
Understanding RecursiveIteratorIterator in PHP
Introduction
RecursiveIteratorIterator is a concrete iterator that implements tree traversal. It enables programmers to traverse container objects that implement the RecursiveIterator interface, allowing for looping over nodes in an ordered tree of objects.
Difference between IteratorIterator and RecursiveIteratorIterator
IteratorIterator is also a concrete iterator, but it performs linear traversal on any Traversable. In contrast, RecursiveIteratorIterator requires a RecursiveIterator to traverse a tree. It exposes its sub-iterator (the currently active iterator) through the getInnerIterator() method, while IteratorIterator exposes its main iterator through the same method.
Technical Differences
- RecursiveIteratorIterator takes a RecursiveIterator as input, while IteratorIterator takes any Traversable.
- RecursiveIteratorIterator has knowledge of parent and children nodes, while IteratorIterator does not.
- RecursiveIteratorIterator maintains a stack of iterators and knows the active sub-iterator, while IteratorIterator does not need a stack.
- RecursiveIteratorIterator has more methods than IteratorIterator.
Traversing a Directory Tree Example
To illustrate the use of these iterators, let's consider a directory tree:
tree ├── dirA │ ├── dirB │ │ └── fileD │ ├── fileB │ └── fileC └── fileA
- Using IteratorIterator:
$dir = new DirectoryIterator('tree'); foreach ($dir as $file) { echo " ├ $file" . PHP_EOL; }
Output:
├ . ├ .. ├ dirA ├ fileA
- Using RecursiveIteratorIterator:
$dir = new RecursiveDirectoryIterator('tree'); $files = new RecursiveIteratorIterator($dir); foreach ($files as $file) { echo " ├ $file" . PHP_EOL; }
Output:
├ tree\. ├ tree\.. ├ tree\dirA\. ├ tree\dirA\.. ├ tree\dirA\dirB\. ├ tree\dirA\dirB\.. ├ tree\dirA\dirB\fileD ├ tree\dirA\fileB ├ tree\dirA\fileC ├ tree\fileA
Customizing Traversal
RecursiveIteratorIterator provides recursion modes that control the order in which nodes are traversed:
- LEAVES_ONLY: List only files
- SELF_FIRST: List directories first, then files
- CHILD_FIRST: List files first, then directories
Conclusion
RecursiveIteratorIterator provides powerful capabilities for traversing tree-like structures in PHP. Its traversal modes offer flexibility, and its meta-information allows for advanced customization of the iteration process.
The above is the detailed content of What are the differences between `IteratorIterator` and `RecursiveIteratorIterator` in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










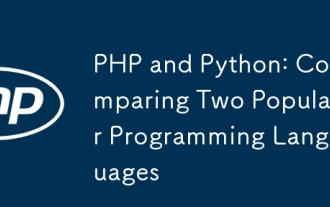
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
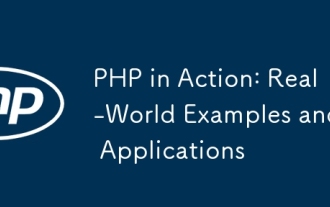
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
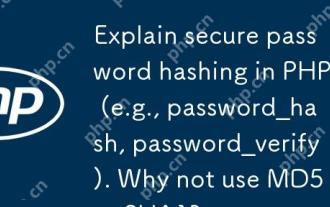
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
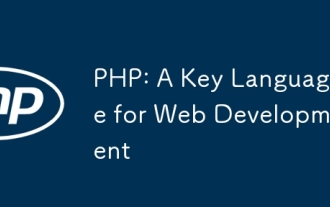
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
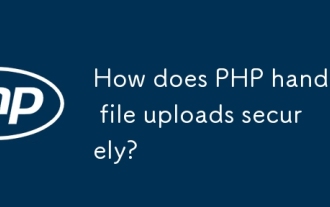
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
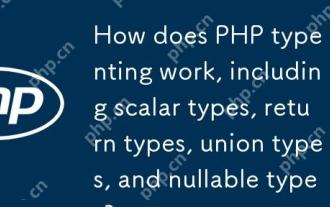
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
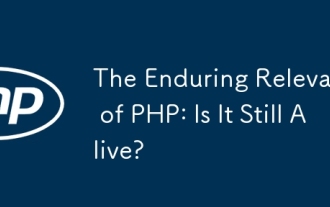
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
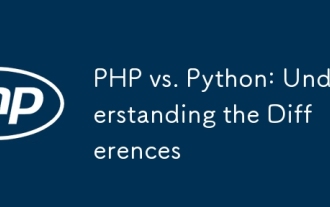
PHP and Python each have their own advantages, and the choice should be based on project requirements. 1.PHP is suitable for web development, with simple syntax and high execution efficiency. 2. Python is suitable for data science and machine learning, with concise syntax and rich libraries.
