


How can you efficiently map one-to-many and many-to-many database relationships to Go structs?
Efficiently Mapping One-to-Many and Many-to-Many Database Relationships to Structs in Go
When working with complex database relationships, mapping them efficiently to Go structs is crucial for maintaining performance and code maintainability. Here are some approaches and their considerations:
Approach 1: Select All Items, Then Select Tags Per Item
This approach is straightforward but inefficient, as it requires separate queries for each item to fetch its related tags. While it may work well for small datasets, it becomes costly for larger ones.
Approach 2: Construct SQL Join and Loop Through Rows Manually
This approach uses a single database query with a join to retrieve related data. While it eliminates the performance hit of multiple queries, it can be cumbersome to develop and maintain, especially for complex queries with multiple joins.
Approach 3: PostgreSQL Array Aggregators and GROUP BY
This approach leverages PostgreSQL array aggregators to group and aggregate related data into arrays. While it's not a direct solution for mapping to Go structs, it can significantly improve performance for large datasets.
Alternative Solution: Utilizing a Postgres View
An alternative approach that addresses the limitations of the previous methods involves creating a Postgres view that returns the desired data structure. The view can perform the necessary joins and aggregations, allowing for efficient retrieval of related data.
In the provided example, the following SQL can create a view named item_tags:
create view item_tags as select id, ( select array_to_json(array_agg(row_to_json(taglist.*))) as array_to_json from ( select tag.name, tag.id from tag where item_id = item.id ) taglist ) as tags from item ;
To map the view to a Go struct, you can execute the following query and unmarshal the result:
type Item struct { ID int Tags []Tag } type Tag struct { ID int Name string } func main() { // Execute the query to fetch the data from the view rows, err := sql.Query("select row_to_json(row)\nfrom ( select * from item_tags\n) row;") if err != nil { // Handle error } // Iterate through the rows and unmarshal the data for rows.Next() { var item Item var data []byte if err := rows.Scan(&data); err != nil { // Handle error } if err := json.Unmarshal(data, &item); err != nil { // Handle error } fmt.Println(item) } }
This approach provides the following benefits:
- Efficient data retrieval: The view can perform the necessary joins and aggregations, resulting in a single efficient query.
- Easy mapping to Go structs: By returning the data in a JSON format, the mapping to Go structs becomes straightforward using the json.Unmarshal function.
- Maintainability: The database logic is encapsulated in the view, making it easier to maintain and reuse across different applications.
The above is the detailed content of How can you efficiently map one-to-many and many-to-many database relationships to Go structs?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


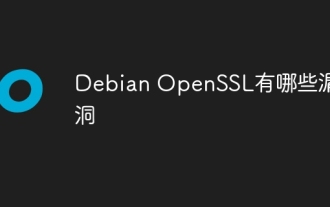
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
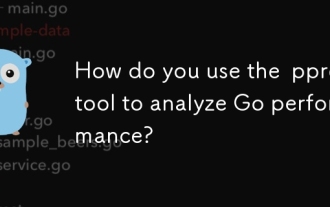
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
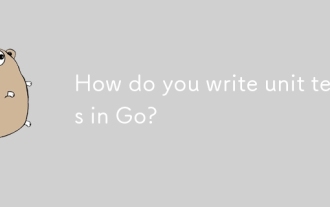
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
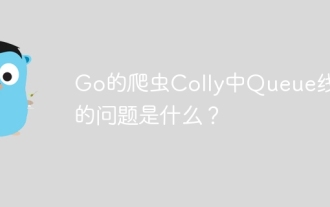
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
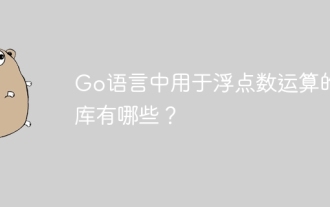
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
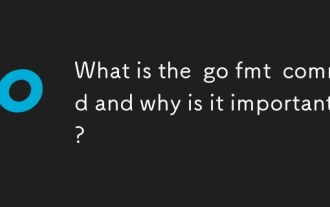
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
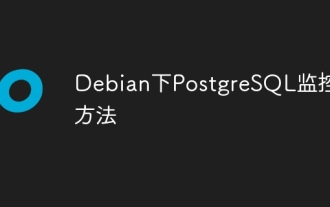
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
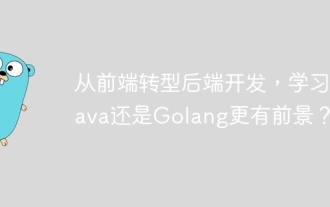
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
