


How to Invoke Python Functions from Node.js: A Comprehensive Guide
Invoking Python Functions from Node.js: A Comprehensive Guide
In this digital age, many applications leverage both Python and Node.js for their diverse capabilities. Python excels in machine learning, while Node.js excels in web development. To harness the power of these technologies in tandem, it becomes crucial to know how to invoke Python functions from Node.js.
Solution
One effective approach is to utilize the "child_process" module that comes bundled with Node.js. This module empowers developers to create subprocesses and interact with external programs.
Step 1: Spawn a Python Process
To execute a Python function from Node.js, you can use the spawn() function within the "child_process" module:
1 2 |
|
In Python, it's essential to import the sys module and access arguments passed from Node.js through sys.argv.
Step 2: Sending Data to Python
To transfer data to the Python script, utilize the stdout stream:
1 |
|
Step 3: Receiving Data from Python
In Node.js, you can listen for data returned from Python through the 'data' event on the stdout stream:
1 2 3 |
|
Customizing Function Invocation
By passing multiple arguments to the Python script via spawn(), you can control which function is executed dynamically. This flexibility allows you to create Python functions tailored to specific arguments.
Conclusion
By following these steps, you can seamlessly invoke Python functions from Node.js applications, unlocking the potential of machine learning integration for your web projects. The "child_process" module provides a robust mechanism for communication between these two languages, empowering developers to leverage the best of both worlds.
The above is the detailed content of How to Invoke Python Functions from Node.js: A Comprehensive Guide. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










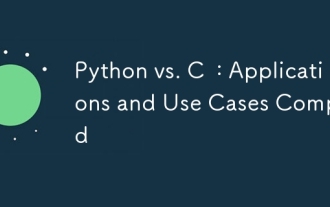
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
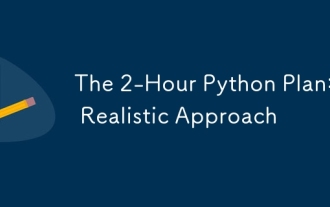
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
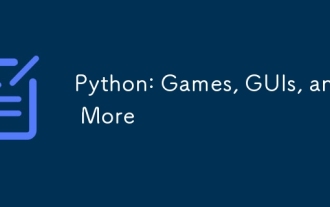
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
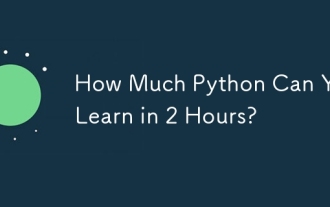
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
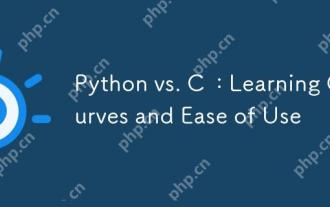
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
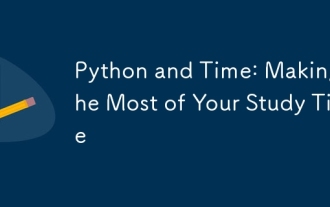
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
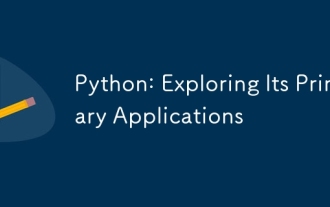
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
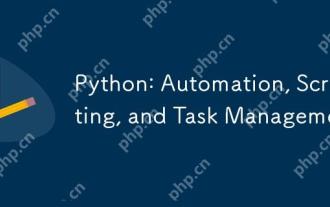
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
