


How to Pinpoint Unhandled Exceptions: A Deep Dive into Custom Exceptions and Macros
Unveiling the Source of Unhandled Exceptions
When exceptions arise during program execution, tracing their origin can be crucial for troubleshooting. In the absence of explicit code line information embedded in the exception message, unhandled and external exceptions can leave developers in the dark.
A Deeper Dive into Custom Exceptions and Macros
To address this challenge, a robust solution involves creating a custom exception class and leveraging macros.
The my_exception class extends the std::runtime_error class and incorporates an additional msg member to store the exception message. The constructor of this class constructs the message by concatenating the source file, line number, and the original exception argument.
Next, the throw_line macro simplifies the process of throwing an exception with line information. It takes an argument representing the exception message and automatically adds the file and line number details to the my_exception object.
Putting it into Action
Consider the following code snippet:
void f() { throw_line("Oh no!"); } int main() { try { f(); } catch (const std::runtime_error &ex) { std::cout << ex.what() << std::endl; } }
When an exception is thrown within the f function, the throw_line macro provides both the exception message ("Oh no!") and the line number where it occurred. This information is accessible through the my_exception object's what() method.
By utilizing this approach, developers gain a valuable tool for precisely identifying the source of exceptions, even when they originate from unhandled or external sources.
The above is the detailed content of How to Pinpoint Unhandled Exceptions: A Deep Dive into Custom Exceptions and Macros. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


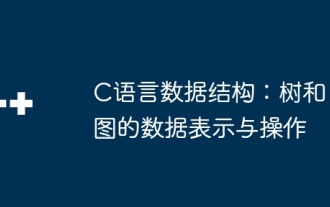
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
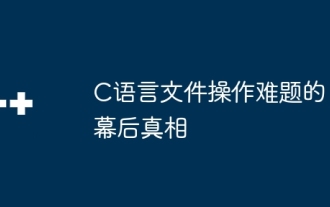
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
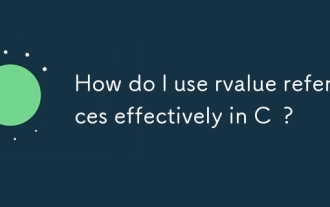
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
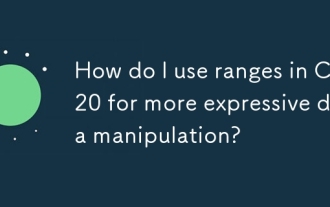
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
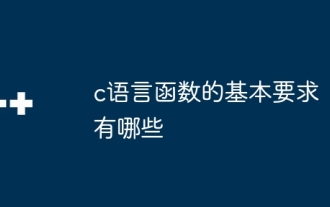
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
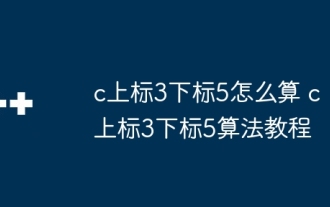
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
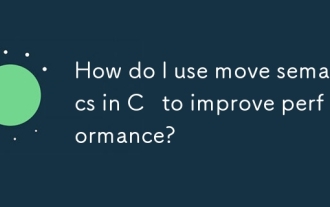
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
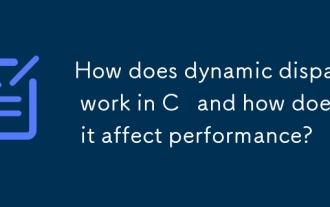
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
