


How Can I Efficiently Apply Vendor Prefixes to CSS Properties with JavaScript?
Nov 18, 2024 pm 07:32 PMSetting Vendor-Prefixed CSS using Javascript
Manually applying vendor prefixes to CSS properties can be tedious. The provided code snippet demonstrates this process for the transform property:
var transform = 'translate3d(0,0,0)'; elem.style.webkitTransform = transform; elem.style.mozTransform = transform; elem.style.msTransform = transform; elem.style.oTransform = transform;
Is there a more efficient way to achieve this, preferably with a single line of JavaScript?
Solution
While there isn't a known library that performs this task, creating a custom function is straightforward since vendor prefixes are consistent in their syntax and names.
function setVendor(element, property, value) { element.style["webkit" + property] = value; element.style["moz" + property] = value; element.style["ms" + property] = value; element.style["o" + property] = value; }
This function can then be used to set vendor-prefixed properties:
setVendor(elem, 'transform', 'translate3d(0,0,0)');
The above is the detailed content of How Can I Efficiently Apply Vendor Prefixes to CSS Properties with JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
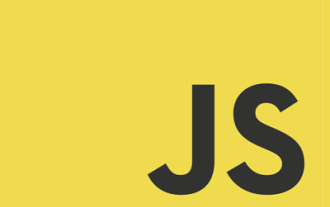
Create a JavaScript Contact Form With the Smart Forms Framework
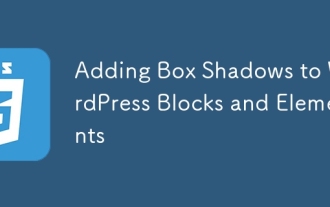
Adding Box Shadows to WordPress Blocks and Elements
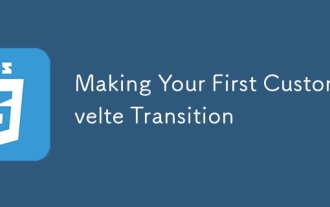
Making Your First Custom Svelte Transition
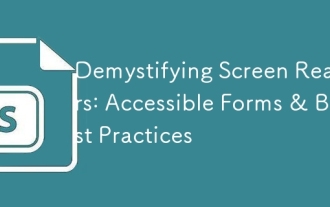
Demystifying Screen Readers: Accessible Forms & Best Practices
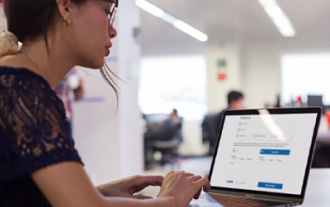
Comparing the 5 Best PHP Form Builders (And 3 Free Scripts)
