


How Can I Efficiently Create a Single Dictionary from a CSV File of Key-Value Pairs?
Creating a Unified Dictionary from a CSV File Consisting of Unique Key-Value Pairs
When handling CSV files, one common task is to create dictionaries where each row represents a unique key-value pair. While the 'csv.DictReader' class provides a convenient way to iterate through such files row-by-row, creating a unified dictionary requires a different approach.
The provided code attempts to construct a dictionary within the nested loop, leading to the 'ValueError' due to attempting to unpack multiple values with only two expected. To address this issue, the syntax should be slightly modified:
Python 3:
import csv with open('coors.csv', mode='r') as infile: reader = csv.reader(infile) with open('coors_new.csv', mode='w') as outfile: writer = csv.writer(outfile) mydict = {rows[0]: rows[1] for rows in reader}
Python 2:
import csv with open('coors.csv', mode='r') as infile: reader = csv.reader(infile) with open('coors_new.csv', mode='w') as outfile: writer = csv.writer(outfile) mydict = dict((rows[0], rows[1]) for rows in reader)
This revised syntax correctly iterates through the CSV file, extracting the key from the first column and the value from the second column for each row. The result is a single dictionary where the keys are unique and sourced from the first column, and the values are sourced from the second column. This approach consolidates all key-value pairs into a comprehensive dictionary.
The above is the detailed content of How Can I Efficiently Create a Single Dictionary from a CSV File of Key-Value Pairs?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
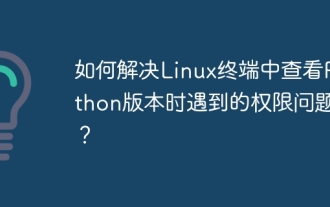
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
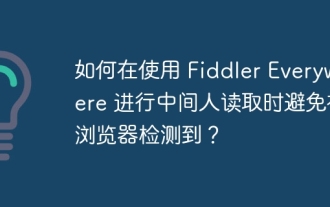
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
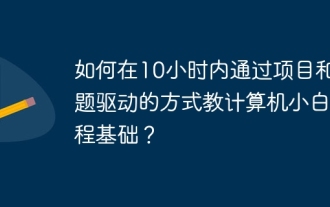
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
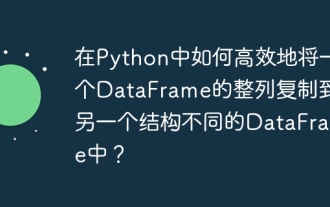
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
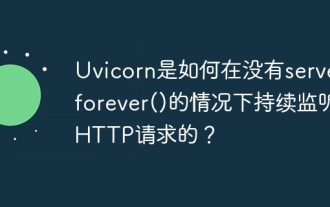
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
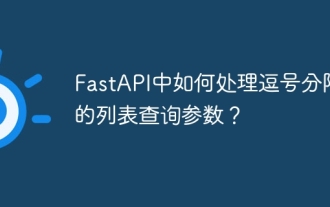
Fastapi ...
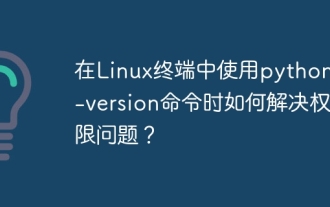
Using python in Linux terminal...
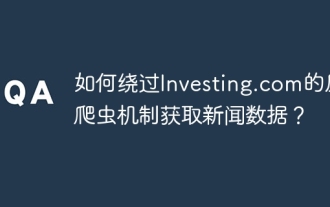
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
