


Devtools Startup Ideas: Building an AI-Powered Debugging Assistant With Code Samples!
I am starting a new series. It focuses on giving devtools ideas to promising founders. These founders are looking to get into the founder space. I have been doing a lot of research on this topic, and will be taking each idea one by one. Giving a foundational overview of what is needed to get started in the business.
What Problem Can Your Startup Solve?
Debugging is one of the most challenging and time-consuming tasks for developers. Spending hours trying to make sense of error messages is exhausting. Combing through lines of code to find the root cause of issues can lead to developer frustration. This process often results in inefficiency.
Imagine building a tool that intelligently identifies real-time code problems and suggests actionable fixes. Developers willlove you!
This article will take a look at building a startup around the concept of an AI-Powered Debugging Assistant startup. Whether you’re a founder exploring devtools startup ideas or a developer seeking inspiration, this step-by-step guide will help you understand the problem it solves. It also explains the technologies behind it. The guide shows you how to build a basic prototype.
Why Build An AI-Powered Debugging Startup?
Challenges Developers Face During Debugging
Time-Consuming Processes: Developers often spend hours analyzing error messages and tracking down subtle issues.
Complex Codebases: Debugging becomes exponentially harder in large, legacy, or poorly documented codebases.
Limited Tools: Traditional tools provide basic static analysis but lack intelligent, context-aware suggestions.
How AI Helps In Code Debugging
Machine Learning for Context: Understands the code and its context to provide tailored suggestions.
Real-Time Fixes: Offers actionable solutions to detected issues, reducing debugging time.
Automation and Productivity: Enhances developer efficiency through intelligent automation.
How the AI-Powered Debugging Assistant Works
This tool will:
- Analyze Python code for errors and inefficiencies.
- Use OpenAI’s GPT for AI-driven explanations and solutions.
- Provide a simple CLI for easy integration into developer workflows.
Technologies Used:
Python: The programming language for code analysis and backend logic.
OpenAI GPT: A powerful model for generating natural language explanations.
AST (Abstract Syntax Tree): For static code analysis.
Step-by-Step Guide to Building an AI-Powered Debugging Assistant Devtool
Step 1: Set Up the Python Development Environment
First, install the required libraries:
pip install openai
You should see a message like this in your terminal, with a success message at the end.
pip install python-dotenv
Building Out The AI Debugger
For simplicity and modularity, you can organize the code snippets into multiple files based on functionality.
Start out in your main.py file. This file will serve as the entry point for your CLI tool.
import sys import os sys.path.insert(0, os.path.abspath(os.path.dirname(__file__))) from analysis import analyze_code from ai_debugger import debug_with_ai def main(): print("Welcome to THDG's Debugging Assistant!") code_snippet = input("Paste your Python code here:\n") syntax_check, _ = analyze_code(code_snippet) print(f"\nSyntax Analysis: {syntax_check}") if "Syntax Error" not in syntax_check: print("\nGenerating AI Debugging Suggestions...") ai_suggestion = debug_with_ai(code_snippet) print("\nAI Suggestion:") print(ai_suggestion) else: print("\nFix the syntax errors before generating AI suggestions.") if __name__ == "__main__": main()
Sometimes, the Python interpreter does not have the current directory in its path. This is why we added
import sys import os sys.path.insert(0, os.path.abspath(os.path.dirname(file)))
at the top of main.py to ensure it includes the script’s directory.
Code Analysis Module
Create a file, analysis.py. This file contains logic for static code analysis using the ast module.
import ast def analyze_code(code): try: tree = ast.parse(code) return "Code is valid!", ast.dump(tree, indent=4) except SyntaxError as e: return f"Syntax Error: {e.msg} at line {e.lineno}", None
This snippet parses Python code to check for syntax errors. It returns the error message or a detailed tree representation of the code structure.
AI Debugging Module
Create a file: ai_debugger.py. This file handles integration with OpenAI’s GPT API for AI-generated suggestions.
import sys import os from openai import OpenAI sys.path.insert(0, os.path.abspath(os.path.dirname(__file__))) from dotenv import load_dotenv load_dotenv() client = OpenAI( api_key=os.environ.get("OPENAI_API_KEY") ) def debug_with_ai(code_snippet): """ Accepts a Python code snippet and returns debugging suggestions. """ # Use ChatCompletion API for conversational responses response = client.chat.completions.create( model="gpt-3.5-turbo", messages=[ {"role": "system", "content": "You are an expert Python debugger."}, {"role": "user", "content": f"Debug the following Python code:\n\n{code_snippet}"} ] ) return response['choices'][0]['message']['content']
Setting Up Your Python Environment File
Store reusable constants or settings, such as your openai API keys or other configurations in the .env file.
OPENAI_API_KEY = "your-openai-api-key"
Challenges With Building An AI Assistant
- Token Limits: Large codebases might exceed token limits for GPT. Solution: Split the code into smaller chunks.
- Accuracy of AI Suggestions: AI-generated suggestions are not always accurate. Ensure to tell users to validate recommendations before applying them.
- Integration Complexity: Integrating the tool with popular IDEs may require additional plugins or APIs.
WhereTo Sell An AI Debugger Devtool
If you have considered this devtool idea, you must consider its actual usecases. This AI-powered assistant can be integrated into:
- IDEs like VSCode: Developers can highlight problematic code, right-click, and receive instant debugging suggestions.
- CI/CD Pipelines: Automatically analyze code in pull requests and suggest fixes during reviews.
- Team Collaboration Tools: Offer insights into code issues during pair programming or team debugging sessions.
Next Steps for Founders
If you’re a founder exploring this devtools startup idea, consider making this a more versatile tool by:
- Expanding to Other Languages: Add support for JavaScript, Java, or Go.
Build a Browser Extension: Create a lightweight tool for debugging code on the web.
Enhance User Experience: Develop a visual dashboard for error analysis and fixes.
The future of dev tools is bright, with opportunities to reshape how developers work and collaborate. With the right vision and execution, this idea could be your startup's success story!
This article was curled from The Handy Developers Guide.
The above is the detailed content of Devtools Startup Ideas: Building an AI-Powered Debugging Assistant With Code Samples!. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
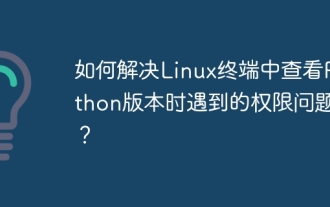
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
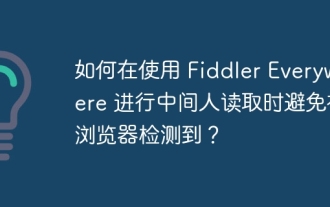
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
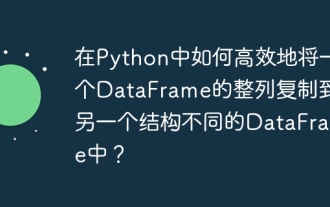
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
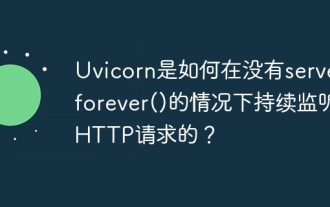
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
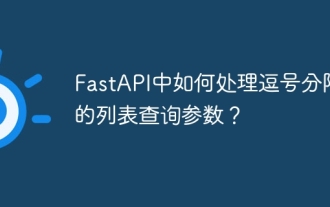
Fastapi ...
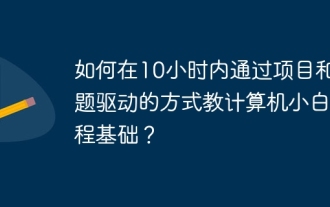
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
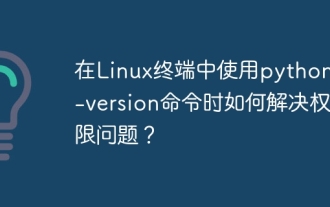
Using python in Linux terminal...
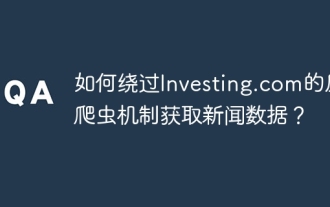
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
