


How to Fix Syntax Errors Caused by Nested Arguments in Python 3 Modules?
Resolving Syntax Errors in Nested Arguments for Python 3 Modules
Issue:
When attempting to compile code into a Python 3 module, users may encounter a syntax error similar to:
SyntaxError: invalid syntax
This error can arise due to the use of nested arguments in function definitions, which were deprecated in Python 3.
Solution:
To rectify this issue, remove tuple parameter unpacking and manually unpack arguments within the function.
For regular functions:
Replace statements like:
<code class="python">def add(self, (sub, pred, obj)): # ...</code>
With:
<code class="python">def add(self, sub_pred_obj): sub, pred, obj = sub_pred_obj # ...</code>
For lambda functions:
Avoid unpacking arguments through assignment; instead, pass and reference the arguments directly:
Replace:
<code class="python">lambda (x, y): (y, x)</code>
With:
<code class="python">lambda xy: (xy[1], xy[0])</code>
Additional Tips:
- Tools such as 2to3, modernize, or futurize can aid in identifying and resolving such issues.
- When porting code from Python 2.x to Python 3.x, it is recommended to use these tools to assist in the conversion process.
The above is the detailed content of How to Fix Syntax Errors Caused by Nested Arguments in Python 3 Modules?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
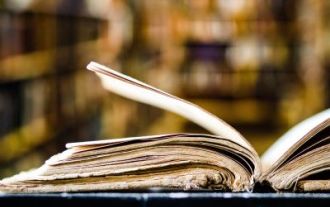
How to Use Python to Find the Zipf Distribution of a Text File
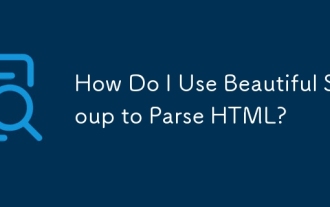
How Do I Use Beautiful Soup to Parse HTML?
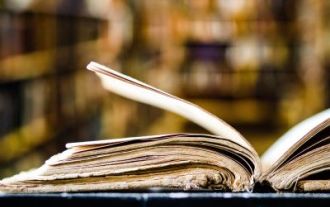
How to Work With PDF Documents Using Python
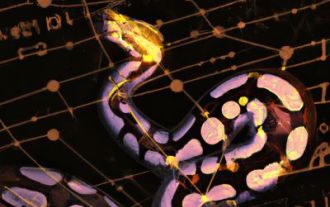
How to Cache Using Redis in Django Applications
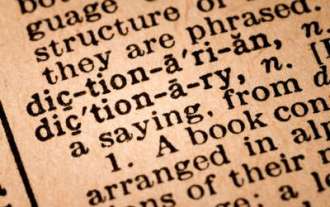
Introducing the Natural Language Toolkit (NLTK)
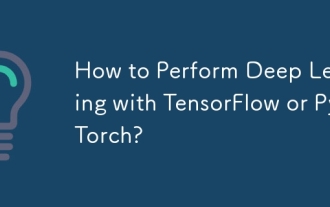
How to Perform Deep Learning with TensorFlow or PyTorch?
