


How Can Java 8\'s `Instant` and `Duration` Simplify Time Difference Calculations?
Calculating Time Differences in Java
Calculating time differences is a common task in programming, and Java provides several methods to achieve this. Suppose you want to determine the difference between two time periods, such as subtracting 16:00:00 from 19:00:00.
For clarity, previous methods involved using the java.util.Date class, which can be cumbersome and error-prone. However, Java 8 introduced cleaner options using Instant and Duration.
An Instant represents a specific point in time, while Duration measures the time interval between two instants. To calculate the difference, you can use the following steps:
import java.time.Duration; import java.time.Instant; // Initialize the starting and ending instants Instant start = Instant.now(); // Insert your code here Instant end = Instant.now(); // Determine the time elapsed Duration timeElapsed = Duration.between(start, end); // Output the results System.out.println("Time taken: " + timeElapsed.toMillis() + " milliseconds");
This approach simplifies time difference calculations by using intuitive classes and methods. The Duration class provides a range of conversion methods, allowing you to easily convert the results to milliseconds, seconds, or minutes as needed.
The above is the detailed content of How Can Java 8\'s `Instant` and `Duration` Simplify Time Difference Calculations?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
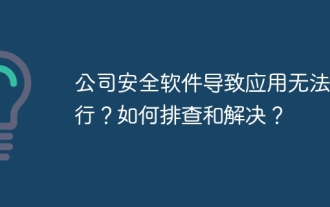
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
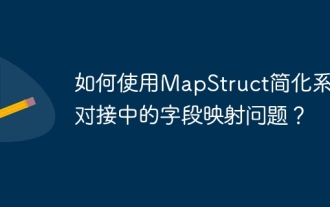
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
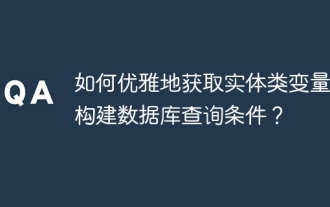
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
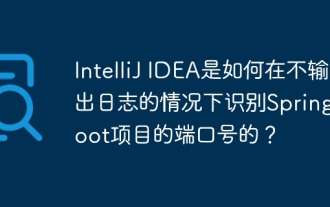
Start Spring using IntelliJIDEAUltimate version...
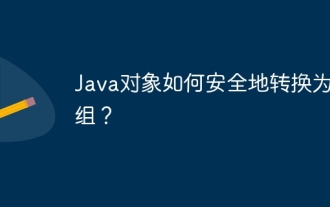
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
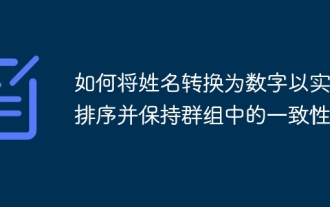
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
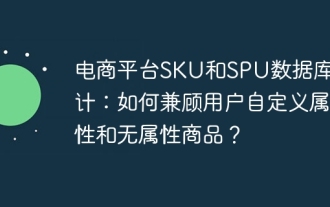
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
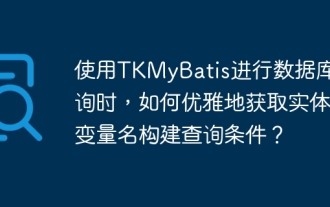
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
