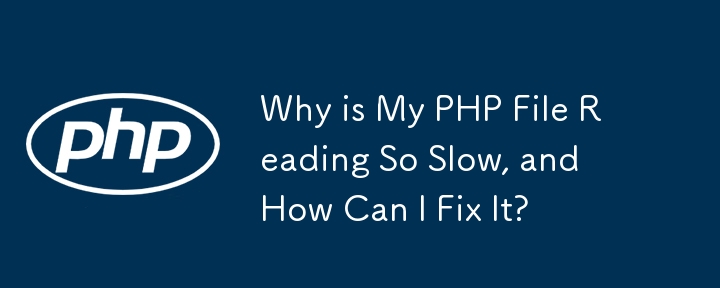
Overcoming File Reading Bottlenecks in PHP
Encountering issues while attempting to read large files in PHP? Fopen may be a potential culprit, but there are various factors that can contribute to read failures. Let's delve into the root of the problem and explore potential solutions.
Troubleshooting Common Errors
-
Timeout: The default timeout setting in PHP scripts is typically around 30 seconds. Reading a large file may exceed this limit, resulting in a timeout error instead of a fopen failure.
-
Memory: Reading the file into an array can consume significant memory, potentially exceeding your script's memory limit. Check your error log for any memory warnings.
-
Permissions: Oversights in file permissions can hinder read operations. Ensure that the file you're attempting to read has the necessary read permissions.
Alternative File Reading Techniques
If these common issues are not applicable, consider utilizing fgets to read the file line-by-line. This approach allows for incremental processing of the file, reducing memory overhead and potential timeout errors. Here's an example:
$handle = fopen("/tmp/uploadfile.txt", "r") or die("Couldn't get handle");
if ($handle) {
while (!feof($handle)) {
$buffer = fgets($handle, 4096);
// Process buffer here..
}
fclose($handle);
}
Copy after login
Additional Considerations
-
File Path: Double-check the path to the file relative to the script's execution location. Absolute paths are recommended for accuracy.
-
File Format: If the file contains non-ASCII characters, the PHP version and your environment's character set may impact reading functionality.
-
Multithreading: PHP lacks native multithreading support, so parallel file processing is not directly available. Consider breaking up the reading process into smaller chunks or using external libraries for multithreading capabilities.
The above is the detailed content of Why is My PHP File Reading So Slow, and How Can I Fix It?. For more information, please follow other related articles on the PHP Chinese website!