


How Can I Simulate Function Pointers in Java Using Anonymous Inner Classes or Lambda Expressions?
Functional Interfaces as Function Pointers in Java
In Java, where function pointers are unavailable, anonymous inner classes or lambda expressions serve as suitable alternatives.
Anonymous Inner Classes
Imagine you need methods that perform similar actions but vary slightly in a single line of computation. To implement this using an anonymous inner class:
- Define an interface containing the function you wish to encapsulate.
interface StringFunction { int func(String param); }
- Create a method that accepts an instance of your interface as a parameter.
public void takingMethod(StringFunction sf) { int i = sf.func("my string"); // Operations... }
- Invoke the method using an anonymous inner class:
ref.takingMethod(new StringFunction() { public int func(String param) { // Implementation... } });
Lambda Expressions (Java 8 )
Syntactically simpler, you can utilize lambda expressions to achieve the same result:
ref.takingMethod(param -> { // Implementation... });
By utilizing anonymous inner classes or lambda expressions, you can create function pointers in Java, enhancing code reusability and flexibility.
The above is the detailed content of How Can I Simulate Function Pointers in Java Using Anonymous Inner Classes or Lambda Expressions?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










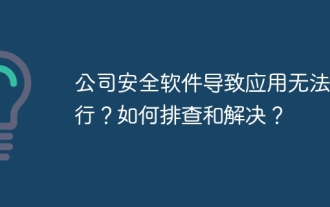
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
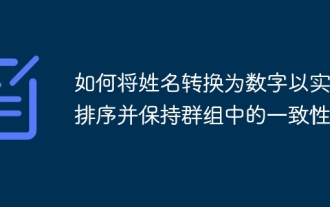
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
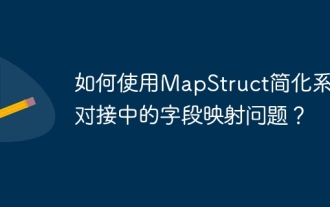
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
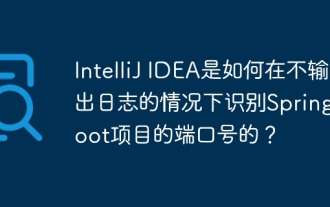
Start Spring using IntelliJIDEAUltimate version...
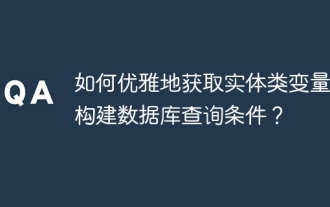
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
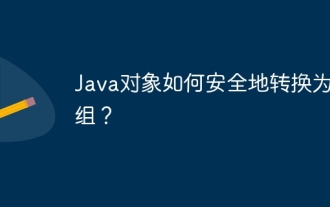
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
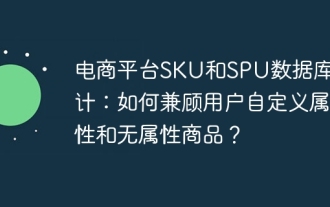
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
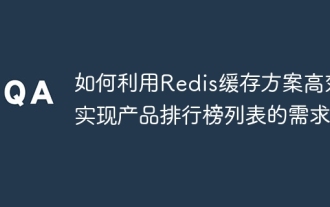
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
