


Why Can\'t I Directly Replace Characters in a Python String Using Index Assignment?
Unable to Replace Characters in String Using Index Assignment
Consider the following code snippet, which attempts to replace semicolons with colons at specified positions within a string:
for i in range(0,len(line)): if (line[i]==";" and i in rightindexarray): line[i]=":"
However, this code fails with a TypeError, indicating that str objects do not support item assignment. This is because strings in Python are immutable, meaning that their contents cannot be directly modified.
Overcoming the Issue
To replace characters within a string, you can use the replace() method:
line = line.replace(';', ':')
This method replaces all occurrences of the specified character (';') with the new character (':').
Selective Replacement
If you only want to replace certain characters, you can use string slicing to isolate the section of the string you want to modify:
line = line[:10].replace(';', ':') + line[10:]
This code will replace all semicolons in the first 10 characters of the string.
Example
Using the code above, the string "Hei der! ; Hello there ;!;", with rightindexarray containing the indices of desired replacements ([3, 13]), would convert to "Hei der! : Hello there :!;".
The above is the detailed content of Why Can\'t I Directly Replace Characters in a Python String Using Index Assignment?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


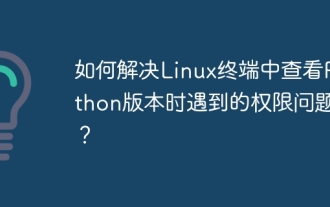
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
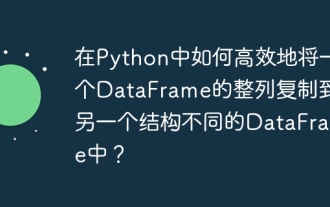
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
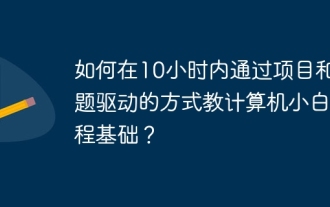
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
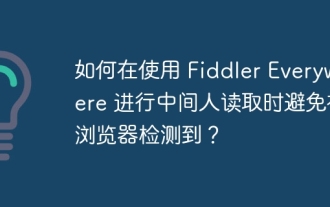
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
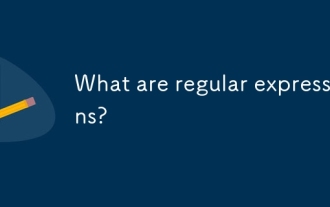
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
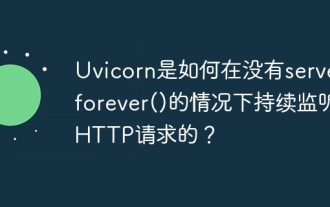
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
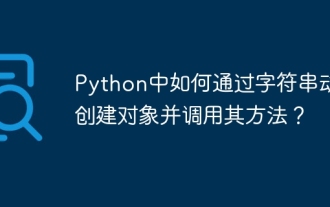
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
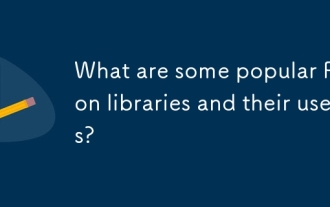
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
