How do I convert a UNIX Epoch Time string into a Java Date object?
Converting UNIX Epoch Time to Java Date Object
In Java, converting a UNIX Epoch time (represented as a string) into a Date object requires a two-step process.
Step 1: Parsing the Epoch Time
The UNIX Epoch time is typically expressed as a string representing the number of seconds since the epoch (January 1, 1970). To parse this string as a long integer, use the Long.parseLong() method, as seen in the following code:
String date = "1081157732"; long epochTime = Long.parseLong(date);
Step 2: Converting Seconds to Milliseconds
Java's Date constructor expects the epoch time to be specified in milliseconds. Therefore, we need to convert the parsed seconds into milliseconds. For this, we can multiply the epochTime by 1000:
long millisecondEpochTime = epochTime * 1000;
Creating the Date Object
Finally, we can create a Date object using the converted millisecondEpochTime:
Date expiry = new Date(millisecondEpochTime);
And that's how you convert a UNIX Epoch time string into a Java Date object. Enjoy coding!
The above is the detailed content of How do I convert a UNIX Epoch Time string into a Java Date object?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










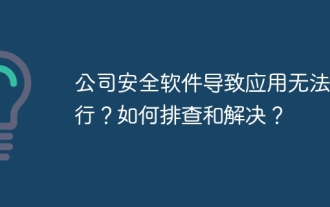
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
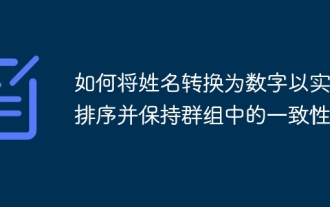
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
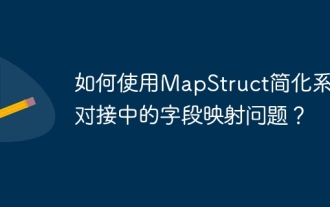
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
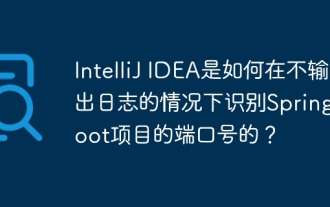
Start Spring using IntelliJIDEAUltimate version...
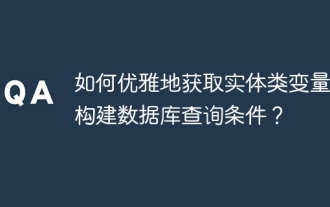
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
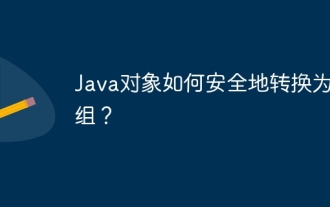
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
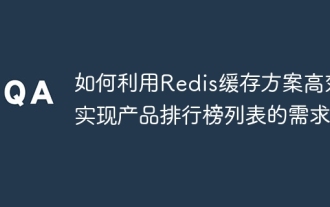
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
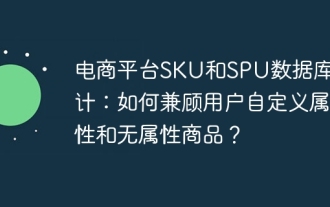
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
