How can I split a string at regular intervals in Java?
Splitting a String at Regular Intervals in Java
In the realm of string manipulation, there may come instances where you desire to split a string into segments based on a specified character count interval. This article addresses how to achieve this task effectively in Java using a combination of regular expressions and the Arrays class.
To illustrate, let's consider the goal of dividing the string "foobarspam" into chunks of three characters each: "foo", "bar", and "spam". In JavaScript, this can be accomplished concisely with the following code:
1 |
|
Java Implementation
To achieve this in Java, we utilize a slightly more elaborate approach that leverages regular expressions and the split() method:
1 2 |
|
This code employs a regular expression within the split() method to specify the desired splitting criteria. Let's break down the expression:
- (?<= matches an empty string that is preceded by (<=):
- G represents the last match in the string.
- .... matches three characters.
By combining these elements, the expression effectively matches empty strings that occur after every three characters in the string.
The output of this code is as follows:
1 |
|
The string has been successfully split into segments of three characters each, providing the desired result.
The above is the detailed content of How can I split a string at regular intervals in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










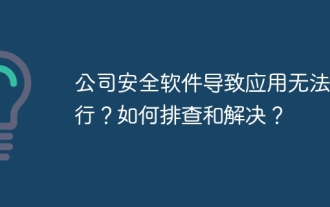
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
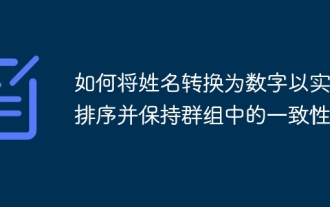
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
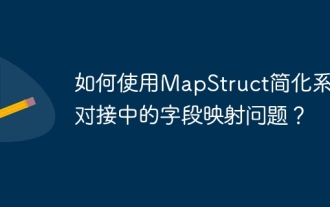
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
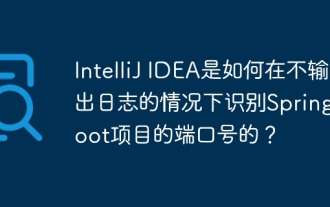
Start Spring using IntelliJIDEAUltimate version...
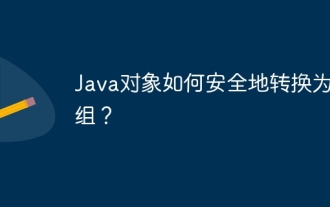
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
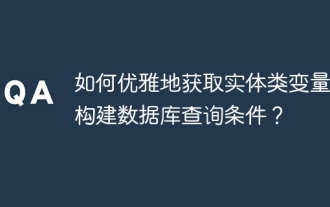
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
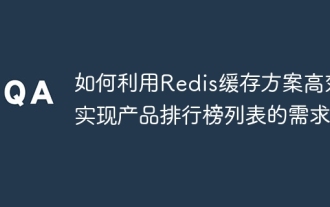
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
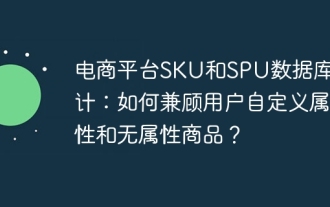
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
